diff options
Diffstat (limited to 'widget/src/pane_grid.rs')
-rw-r--r-- | widget/src/pane_grid.rs | 78 |
1 files changed, 64 insertions, 14 deletions
diff --git a/widget/src/pane_grid.rs b/widget/src/pane_grid.rs index 4473119d..9d4dda25 100644 --- a/widget/src/pane_grid.rs +++ b/widget/src/pane_grid.rs @@ -1,8 +1,54 @@ -//! Let your users split regions of your application and organize layout dynamically. +//! Pane grids let your users split regions of your application and organize layout dynamically. //! //! 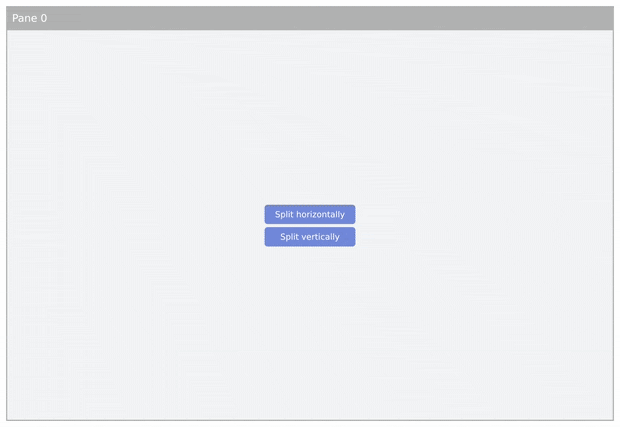 //! +//! This distribution of space is common in tiling window managers (like +//! [`awesome`](https://awesomewm.org/), [`i3`](https://i3wm.org/), or even +//! [`tmux`](https://github.com/tmux/tmux)). +//! +//! A [`PaneGrid`] supports: +//! +//! * Vertical and horizontal splits +//! * Tracking of the last active pane +//! * Mouse-based resizing +//! * Drag and drop to reorganize panes +//! * Hotkey support +//! * Configurable modifier keys +//! * [`State`] API to perform actions programmatically (`split`, `swap`, `resize`, etc.) +//! //! # Example +//! ```no_run +//! # mod iced { pub mod widget { pub use iced_widget::*; } pub use iced_widget::Renderer; pub use iced_widget::core::*; } +//! # pub type Element<'a, Message> = iced_widget::core::Element<'a, Message, iced_widget::Theme, iced_widget::Renderer>; +//! # +//! use iced::widget::{pane_grid, text}; +//! +//! struct State { +//! panes: pane_grid::State<Pane>, +//! } +//! +//! enum Pane { +//! SomePane, +//! AnotherKindOfPane, +//! } +//! +//! enum Message { +//! PaneDragged(pane_grid::DragEvent), +//! PaneResized(pane_grid::ResizeEvent), +//! } +//! +//! fn view(state: &State) -> Element<'_, Message> { +//! pane_grid(&state.panes, |pane, state, is_maximized| { +//! pane_grid::Content::new(match state { +//! Pane::SomePane => text("This is some pane"), +//! Pane::AnotherKindOfPane => text("This is another kind of pane"), +//! }) +//! }) +//! .on_drag(Message::PaneDragged) +//! .on_resize(10, Message::PaneResized) +//! .into() +//! } +//! ``` //! The [`pane_grid` example] showcases how to use a [`PaneGrid`] with resizing, //! drag and drop, and hotkey support. //! @@ -68,14 +114,18 @@ const THICKNESS_RATIO: f32 = 25.0; /// * Configurable modifier keys /// * [`State`] API to perform actions programmatically (`split`, `swap`, `resize`, etc.) /// -/// ## Example -/// +/// # Example /// ```no_run -/// # use iced_widget::{pane_grid, text}; -/// # -/// # type PaneGrid<'a, Message> = iced_widget::PaneGrid<'a, Message>; +/// # mod iced { pub mod widget { pub use iced_widget::*; } pub use iced_widget::Renderer; pub use iced_widget::core::*; } +/// # pub type Element<'a, Message> = iced_widget::core::Element<'a, Message, iced_widget::Theme, iced_widget::Renderer>; /// # -/// enum PaneState { +/// use iced::widget::{pane_grid, text}; +/// +/// struct State { +/// panes: pane_grid::State<Pane>, +/// } +/// +/// enum Pane { /// SomePane, /// AnotherKindOfPane, /// } @@ -85,17 +135,17 @@ const THICKNESS_RATIO: f32 = 25.0; /// PaneResized(pane_grid::ResizeEvent), /// } /// -/// let (mut state, _) = pane_grid::State::new(PaneState::SomePane); -/// -/// let pane_grid = -/// PaneGrid::new(&state, |pane, state, is_maximized| { +/// fn view(state: &State) -> Element<'_, Message> { +/// pane_grid(&state.panes, |pane, state, is_maximized| { /// pane_grid::Content::new(match state { -/// PaneState::SomePane => text("This is some pane"), -/// PaneState::AnotherKindOfPane => text("This is another kind of pane"), +/// Pane::SomePane => text("This is some pane"), +/// Pane::AnotherKindOfPane => text("This is another kind of pane"), /// }) /// }) /// .on_drag(Message::PaneDragged) -/// .on_resize(10, Message::PaneResized); +/// .on_resize(10, Message::PaneResized) +/// .into() +/// } /// ``` #[allow(missing_debug_implementations)] pub struct PaneGrid< |