diff options
Diffstat (limited to 'src/widget')
-rw-r--r-- | src/widget/button.rs | 284 | ||||
-rw-r--r-- | src/widget/checkbox.rs | 199 | ||||
-rw-r--r-- | src/widget/column.rs | 224 | ||||
-rw-r--r-- | src/widget/image.rs | 180 | ||||
-rw-r--r-- | src/widget/panel.rs | 94 | ||||
-rw-r--r-- | src/widget/progress_bar.rs | 106 | ||||
-rw-r--r-- | src/widget/radio.rs | 207 | ||||
-rw-r--r-- | src/widget/row.rs | 219 | ||||
-rw-r--r-- | src/widget/slider.rs | 245 | ||||
-rw-r--r-- | src/widget/text.rs | 218 |
10 files changed, 0 insertions, 1976 deletions
diff --git a/src/widget/button.rs b/src/widget/button.rs deleted file mode 100644 index d2ea70e4..00000000 --- a/src/widget/button.rs +++ /dev/null @@ -1,284 +0,0 @@ -//! Allow your users to perform actions by pressing a button. -//! -//! A [`Button`] has some local [`State`] and a [`Class`]. -//! -//! [`Button`]: struct.Button.html -//! [`State`]: struct.State.html -//! [`Class`]: enum.Class.html - -use crate::input::{mouse, ButtonState}; -use crate::{ - Align, Element, Event, Hasher, Layout, MouseCursor, Node, Point, Rectangle, - Style, Widget, -}; - -use std::hash::Hash; - -/// A generic widget that produces a message when clicked. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`button::Renderer`] trait. -/// -/// [`Widget`]: ../trait.Widget.html -/// [`button::Renderer`]: trait.Renderer.html -/// -/// # Example -/// -/// ``` -/// use iced::{button, Button}; -/// -/// pub enum Message { -/// ButtonClicked, -/// } -/// -/// let state = &mut button::State::new(); -/// -/// Button::new(state, "Click me!") -/// .on_press(Message::ButtonClicked); -/// ``` -/// -///  -pub struct Button<'a, Message> { - state: &'a mut State, - /// The label of the button. - pub label: String, - class: Class, - /// The message to produce when the button is pressed - pub on_press: Option<Message>, - style: Style, -} - -impl<'a, Message> std::fmt::Debug for Button<'a, Message> -where - Message: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Button") - .field("state", &self.state) - .field("label", &self.label) - .field("class", &self.class) - .field("on_press", &self.on_press) - .field("style", &self.style) - .finish() - } -} - -impl<'a, Message> Button<'a, Message> { - /// Creates a new [`Button`] with some local [`State`] and the given label. - /// - /// The default [`Class`] of a new [`Button`] is [`Class::Primary`]. - /// - /// [`Button`]: struct.Button.html - /// [`State`]: struct.State.html - /// [`Class`]: enum.Class.html - /// [`Class::Primary`]: enum.Class.html#variant.Primary - pub fn new(state: &'a mut State, label: &str) -> Self { - Button { - state, - label: String::from(label), - class: Class::Primary, - on_press: None, - style: Style::default().min_width(100), - } - } - - /// Sets the width of the [`Button`] in pixels. - /// - /// [`Button`]: struct.Button.html - pub fn width(mut self, width: u16) -> Self { - self.style = self.style.width(width); - self - } - - /// Makes the [`Button`] fill the horizontal space of its container. - /// - /// [`Button`]: struct.Button.html - pub fn fill_width(mut self) -> Self { - self.style = self.style.fill_width(); - self - } - - /// Sets the alignment of the [`Button`] itself. - /// - /// This is useful if you want to override the default alignment given by - /// the parent container. - /// - /// [`Button`]: struct.Button.html - pub fn align_self(mut self, align: Align) -> Self { - self.style = self.style.align_self(align); - self - } - - /// Sets the [`Class`] of the [`Button`]. - /// - /// - /// [`Button`]: struct.Button.html - /// [`Class`]: enum.Class.html - pub fn class(mut self, class: Class) -> Self { - self.class = class; - self - } - - /// Sets the message that will be produced when the [`Button`] is pressed. - /// - /// [`Button`]: struct.Button.html - pub fn on_press(mut self, msg: Message) -> Self { - self.on_press = Some(msg); - self - } -} - -impl<'a, Message, Renderer> Widget<Message, Renderer> for Button<'a, Message> -where - Renderer: self::Renderer, - Message: Copy + std::fmt::Debug, -{ - fn node(&self, _renderer: &mut Renderer) -> Node { - Node::new(self.style.height(50)) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - match event { - Event::Mouse(mouse::Event::Input { - button: mouse::Button::Left, - state, - }) => { - if let Some(on_press) = self.on_press { - let bounds = layout.bounds(); - - match state { - ButtonState::Pressed => { - self.state.is_pressed = - bounds.contains(cursor_position); - } - ButtonState::Released => { - let is_clicked = self.state.is_pressed - && bounds.contains(cursor_position); - - self.state.is_pressed = false; - - if is_clicked { - messages.push(on_press); - } - } - } - } - } - _ => {} - } - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - cursor_position: Point, - ) -> MouseCursor { - renderer.draw( - cursor_position, - layout.bounds(), - self.state, - &self.label, - self.class, - ) - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - } -} - -/// The local state of a [`Button`]. -/// -/// [`Button`]: struct.Button.html -#[derive(Debug, Clone, Copy, PartialEq, Eq, Default)] -pub struct State { - is_pressed: bool, -} - -impl State { - /// Creates a new [`State`]. - /// - /// [`State`]: struct.State.html - pub fn new() -> State { - State::default() - } - - /// Returns whether the associated [`Button`] is currently being pressed or - /// not. - /// - /// [`Button`]: struct.Button.html - pub fn is_pressed(&self) -> bool { - self.is_pressed - } -} - -/// The type of a [`Button`]. -/// -/// 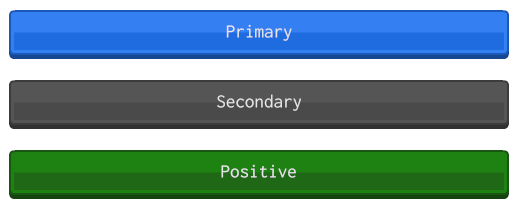 -/// -/// [`Button`]: struct.Button.html -#[derive(Debug, Clone, Copy, PartialEq, Eq)] -pub enum Class { - /// The [`Button`] performs the main action. - /// - /// [`Button`]: struct.Button.html - Primary, - - /// The [`Button`] performs an alternative action. - /// - /// [`Button`]: struct.Button.html - Secondary, - - /// The [`Button`] performs a productive action. - /// - /// [`Button`]: struct.Button.html - Positive, -} - -/// The renderer of a [`Button`]. -/// -/// Your [renderer] will need to implement this trait before being -/// able to use a [`Button`] in your user interface. -/// -/// [`Button`]: struct.Button.html -/// [renderer]: ../../renderer/index.html -pub trait Renderer { - /// Draws a [`Button`]. - /// - /// It receives: - /// * the current cursor position - /// * the bounds of the [`Button`] - /// * the local state of the [`Button`] - /// * the label of the [`Button`] - /// * the [`Class`] of the [`Button`] - /// - /// [`Button`]: struct.Button.html - /// [`State`]: struct.State.html - /// [`Class`]: enum.Class.html - fn draw( - &mut self, - cursor_position: Point, - bounds: Rectangle, - state: &State, - label: &str, - class: Class, - ) -> MouseCursor; -} - -impl<'a, Message, Renderer> From<Button<'a, Message>> - for Element<'a, Message, Renderer> -where - Renderer: self::Renderer, - Message: 'static + Copy + std::fmt::Debug, -{ - fn from(button: Button<'a, Message>) -> Element<'a, Message, Renderer> { - Element::new(button) - } -} diff --git a/src/widget/checkbox.rs b/src/widget/checkbox.rs deleted file mode 100644 index 48400fae..00000000 --- a/src/widget/checkbox.rs +++ /dev/null @@ -1,199 +0,0 @@ -//! Show toggle controls using checkboxes. -use std::hash::Hash; - -use crate::input::{mouse, ButtonState}; -use crate::widget::{text, Column, Row, Text}; -use crate::{ - Align, Color, Element, Event, Hasher, Layout, MouseCursor, Node, Point, - Rectangle, Widget, -}; - -/// A box that can be checked. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`checkbox::Renderer`] trait. -/// -/// [`Widget`]: ../trait.Widget.html -/// [`checkbox::Renderer`]: trait.Renderer.html -/// -/// # Example -/// -/// ``` -/// use iced::{Checkbox, Color}; -/// -/// pub enum Message { -/// CheckboxToggled(bool), -/// } -/// -/// let is_checked = true; -/// -/// Checkbox::new(is_checked, "Toggle me!", Message::CheckboxToggled); -/// ``` -/// -/// 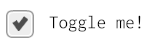 -pub struct Checkbox<Message> { - /// Whether the checkbox is checked or not - pub is_checked: bool, - - /// Function to call when checkbox is toggled to produce a __message__. - /// - /// The function should be provided `true` when the checkbox is checked - /// and `false` otherwise. - pub on_toggle: Box<dyn Fn(bool) -> Message>, - - /// The label of the checkbox - pub label: String, - - /// The color of the label - pub label_color: Option<Color>, -} - -impl<Message> std::fmt::Debug for Checkbox<Message> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Checkbox") - .field("is_checked", &self.is_checked) - .field("label", &self.label) - .field("label_color", &self.label_color) - .finish() - } -} - -impl<Message> Checkbox<Message> { - /// Creates a new [`Checkbox`]. - /// - /// It expects: - /// * a boolean describing whether the [`Checkbox`] is checked or not - /// * the label of the [`Checkbox`] - /// * a function that will be called when the [`Checkbox`] is toggled. - /// It will receive the new state of the [`Checkbox`] and must produce - /// a `Message`. - /// - /// [`Checkbox`]: struct.Checkbox.html - pub fn new<F>(is_checked: bool, label: &str, f: F) -> Self - where - F: 'static + Fn(bool) -> Message, - { - Checkbox { - is_checked, - on_toggle: Box::new(f), - label: String::from(label), - label_color: None, - } - } - - /// Sets the color of the label of the [`Checkbox`]. - /// - /// [`Checkbox`]: struct.Checkbox.html - pub fn label_color<C: Into<Color>>(mut self, color: C) -> Self { - self.label_color = Some(color.into()); - self - } -} - -impl<Message, Renderer> Widget<Message, Renderer> for Checkbox<Message> -where - Renderer: self::Renderer + text::Renderer, -{ - fn node(&self, renderer: &mut Renderer) -> Node { - Row::<(), Renderer>::new() - .spacing(15) - .align_items(Align::Center) - .push(Column::new().width(28).height(28)) - .push(Text::new(&self.label)) - .node(renderer) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - match event { - Event::Mouse(mouse::Event::Input { - button: mouse::Button::Left, - state: ButtonState::Pressed, - }) => { - let mouse_over = layout - .children() - .any(|child| child.bounds().contains(cursor_position)); - - if mouse_over { - messages.push((self.on_toggle)(!self.is_checked)); - } - } - _ => {} - } - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - cursor_position: Point, - ) -> MouseCursor { - let children: Vec<_> = layout.children().collect(); - - let text_bounds = children[1].bounds(); - - text::Renderer::draw( - renderer, - text_bounds, - &self.label, - None, - self.label_color, - text::HorizontalAlignment::Left, - text::VerticalAlignment::Top, - ); - - self::Renderer::draw( - renderer, - cursor_position, - children[0].bounds(), - text_bounds, - self.is_checked, - ) - } - - fn hash_layout(&self, state: &mut Hasher) { - self.label.hash(state); - } -} - -/// The renderer of a [`Checkbox`]. -/// -/// Your [renderer] will need to implement this trait before being -/// able to use a [`Checkbox`] in your user interface. -/// -/// [`Checkbox`]: struct.Checkbox.html -/// [renderer]: ../../renderer/index.html -pub trait Renderer { - /// Draws a [`Checkbox`]. - /// - /// It receives: - /// * the current cursor position - /// * the bounds of the [`Checkbox`] - /// * the bounds of the label of the [`Checkbox`] - /// * whether the [`Checkbox`] is checked or not - /// - /// [`Checkbox`]: struct.Checkbox.html - fn draw( - &mut self, - cursor_position: Point, - bounds: Rectangle, - label_bounds: Rectangle, - is_checked: bool, - ) -> MouseCursor; -} - -impl<'a, Message, Renderer> From<Checkbox<Message>> - for Element<'a, Message, Renderer> -where - Renderer: self::Renderer + text::Renderer, - Message: 'static, -{ - fn from(checkbox: Checkbox<Message>) -> Element<'a, Message, Renderer> { - Element::new(checkbox) - } -} diff --git a/src/widget/column.rs b/src/widget/column.rs deleted file mode 100644 index 831f5b8f..00000000 --- a/src/widget/column.rs +++ /dev/null @@ -1,224 +0,0 @@ -use std::hash::Hash; - -use crate::{ - Align, Element, Event, Hasher, Justify, Layout, MouseCursor, Node, Point, - Style, Widget, -}; - -/// A container that distributes its contents vertically. -/// -/// A [`Column`] will try to fill the horizontal space of its container. -/// -/// [`Column`]: struct.Column.html -#[derive(Default)] -pub struct Column<'a, Message, Renderer> { - style: Style, - spacing: u16, - children: Vec<Element<'a, Message, Renderer>>, -} - -impl<'a, Message, Renderer> std::fmt::Debug for Column<'a, Message, Renderer> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Column") - .field("style", &self.style) - .field("spacing", &self.spacing) - .field("children", &self.children) - .finish() - } -} - -impl<'a, Message, Renderer> Column<'a, Message, Renderer> { - /// Creates an empty [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn new() -> Self { - let mut style = Style::default().fill_width(); - style.0.flex_direction = stretch::style::FlexDirection::Column; - - Column { - style, - spacing: 0, - children: Vec::new(), - } - } - - /// Sets the vertical spacing _between_ elements in pixels. - /// - /// Custom margins per element do not exist in Iced. You should use this - /// method instead! While less flexible, it helps you keep spacing between - /// elements consistent. - pub fn spacing(mut self, px: u16) -> Self { - self.spacing = px; - self - } - - /// Sets the padding of the [`Column`] in pixels. - /// - /// [`Column`]: struct.Column.html - pub fn padding(mut self, px: u16) -> Self { - self.style = self.style.padding(px); - self - } - - /// Sets the width of the [`Column`] in pixels. - /// - /// [`Column`]: struct.Column.html - pub fn width(mut self, width: u16) -> Self { - self.style = self.style.width(width); - self - } - - /// Sets the height of the [`Column`] in pixels. - /// - /// [`Column`]: struct.Column.html - pub fn height(mut self, height: u16) -> Self { - self.style = self.style.height(height); - self - } - - /// Sets the maximum width of the [`Column`] in pixels. - /// - /// [`Column`]: struct.Column.html - pub fn max_width(mut self, max_width: u16) -> Self { - self.style = self.style.max_width(max_width); - self - } - - /// Sets the maximum height of the [`Column`] in pixels. - /// - /// [`Column`]: struct.Column.html - pub fn max_height(mut self, max_height: u16) -> Self { - self.style = self.style.max_height(max_height); - self - } - - /// Sets the alignment of the [`Column`] itself. - /// - /// This is useful if you want to override the default alignment given by - /// the parent container. - /// - /// [`Column`]: struct.Column.html - pub fn align_self(mut self, align: Align) -> Self { - self.style = self.style.align_self(align); - self - } - - /// Sets the horizontal alignment of the contents of the [`Column`] . - /// - /// [`Column`]: struct.Column.html - pub fn align_items(mut self, align: Align) -> Self { - self.style = self.style.align_items(align); - self - } - - /// Sets the vertical distribution strategy for the contents of the - /// [`Column`] . - /// - /// [`Column`]: struct.Column.html - pub fn justify_content(mut self, justify: Justify) -> Self { - self.style = self.style.justify_content(justify); - self - } - - /// Adds an [`Element`] to the [`Column`]. - /// - /// [`Element`]: ../struct.Element.html - /// [`Column`]: struct.Column.html - pub fn push<E>(mut self, child: E) -> Column<'a, Message, Renderer> - where - E: Into<Element<'a, Message, Renderer>>, - { - self.children.push(child.into()); - self - } -} - -impl<'a, Message, Renderer> Widget<Message, Renderer> - for Column<'a, Message, Renderer> -{ - fn node(&self, renderer: &mut Renderer) -> Node { - let mut children: Vec<Node> = self - .children - .iter() - .map(|child| { - let mut node = child.widget.node(renderer); - - let mut style = node.0.style(); - style.margin.bottom = - stretch::style::Dimension::Points(f32::from(self.spacing)); - - node.0.set_style(style); - node - }) - .collect(); - - if let Some(node) = children.last_mut() { - let mut style = node.0.style(); - style.margin.bottom = stretch::style::Dimension::Undefined; - - node.0.set_style(style); - } - - Node::with_children(self.style, children) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - self.children.iter_mut().zip(layout.children()).for_each( - |(child, layout)| { - child - .widget - .on_event(event, layout, cursor_position, messages) - }, - ); - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - cursor_position: Point, - ) -> MouseCursor { - let mut cursor = MouseCursor::OutOfBounds; - - self.children.iter().zip(layout.children()).for_each( - |(child, layout)| { - let new_cursor = - child.widget.draw(renderer, layout, cursor_position); - - if new_cursor != MouseCursor::OutOfBounds { - cursor = new_cursor; - } - }, - ); - - cursor - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - self.spacing.hash(state); - - for child in &self.children { - child.widget.hash_layout(state); - } - } -} - -impl<'a, Message, Renderer> From<Column<'a, Message, Renderer>> - for Element<'a, Message, Renderer> -where - Renderer: 'a, - Message: 'static, -{ - fn from( - column: Column<'a, Message, Renderer>, - ) -> Element<'a, Message, Renderer> { - Element::new(column) - } -} diff --git a/src/widget/image.rs b/src/widget/image.rs deleted file mode 100644 index 1601234e..00000000 --- a/src/widget/image.rs +++ /dev/null @@ -1,180 +0,0 @@ -//! Display images in your user interface. - -use crate::{ - Align, Element, Hasher, Layout, MouseCursor, Node, Point, Rectangle, Style, - Widget, -}; - -use std::hash::Hash; - -/// A frame that displays an image while keeping aspect ratio. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`image::Renderer`] trait. -/// -/// [`Widget`]: ../../core/trait.Widget.html -/// [`image::Renderer`]: trait.Renderer.html -/// -/// # Example -/// -/// ``` -/// use iced::Image; -/// -/// # let my_handle = String::from("some_handle"); -/// let image = Image::new(my_handle); -/// ``` -pub struct Image<I> { - /// The image handle - pub image: I, - source: Option<Rectangle<u16>>, - /// The width of the image - pub width: Option<u16>, - height: Option<u16>, - style: Style, -} - -impl<I> std::fmt::Debug for Image<I> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Image") - .field("source", &self.source) - .field("width", &self.width) - .field("height", &self.height) - .field("style", &self.style) - .finish() - } -} - -impl<I> Image<I> { - /// Creates a new [`Image`] with given image handle. - /// - /// [`Image`]: struct.Image.html - pub fn new(image: I) -> Self { - Image { - image, - source: None, - width: None, - height: None, - style: Style::default(), - } - } - - /// Sets the portion of the [`Image`] to draw. - /// - /// [`Image`]: struct.Image.html - pub fn clip(mut self, source: Rectangle<u16>) -> Self { - self.source = Some(source); - self - } - - /// Sets the width of the [`Image`] boundaries in pixels. - /// - /// [`Image`]: struct.Image.html - pub fn width(mut self, width: u16) -> Self { - self.width = Some(width); - self - } - - /// Sets the height of the [`Image`] boundaries in pixels. - /// - /// [`Image`]: struct.Image.html - pub fn height(mut self, height: u16) -> Self { - self.height = Some(height); - self - } - - /// Sets the alignment of the [`Image`] itself. - /// - /// This is useful if you want to override the default alignment given by - /// the parent container. - /// - /// [`Image`]: struct.Image.html - pub fn align_self(mut self, align: Align) -> Self { - self.style = self.style.align_self(align); - self - } -} - -impl<I, Message, Renderer> Widget<Message, Renderer> for Image<I> -where - Renderer: self::Renderer<I>, - I: Clone, -{ - fn node(&self, renderer: &mut Renderer) -> Node { - renderer.node( - self.style, - &self.image, - self.width, - self.height, - self.source, - ) - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - _cursor_position: Point, - ) -> MouseCursor { - renderer.draw(&self.image, layout.bounds(), self.source); - - MouseCursor::OutOfBounds - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - self.width.hash(state); - self.height.hash(state); - } -} - -/// The renderer of an [`Image`]. -/// -/// Your [renderer] will need to implement this trait before being able to use -/// an [`Image`] in your user interface. -/// -/// [`Image`]: struct.Image.html -/// [renderer]: ../../renderer/index.html -pub trait Renderer<I> { - /// Creates a [`Node`] with the given [`Style`] for the provided [`Image`] - /// and its size. - /// - /// You should probably keep the original aspect ratio, if possible. - /// - /// [`Node`]: ../../struct.Node.html - /// [`Style`]: ../../struct.Style.html - /// [`Image`]: struct.Image.html - fn node( - &mut self, - style: Style, - image: &I, - width: Option<u16>, - height: Option<u16>, - source: Option<Rectangle<u16>>, - ) -> Node; - - /// Draws an [`Image`]. - /// - /// It receives: - /// * the bounds of the [`Image`] - /// * the handle of the loaded [`Image`] - /// * the portion of the image to draw. If not specified, the entire image - /// should be drawn. - /// - /// [`Image`]: struct.Image.html - fn draw( - &mut self, - image: &I, - bounds: Rectangle<f32>, - source: Option<Rectangle<u16>>, - ); -} - -impl<'a, I, Message, Renderer> From<Image<I>> for Element<'a, Message, Renderer> -where - Renderer: self::Renderer<I>, - I: Clone + 'a, -{ - fn from(image: Image<I>) -> Element<'a, Message, Renderer> { - Element::new(image) - } -} diff --git a/src/widget/panel.rs b/src/widget/panel.rs deleted file mode 100644 index d43d6fb6..00000000 --- a/src/widget/panel.rs +++ /dev/null @@ -1,94 +0,0 @@ -use std::hash::Hash; - -use crate::graphics::{Point, Rectangle}; -use crate::ui::core::{ - Event, Hasher, Layout, MouseCursor, Node, Style, Widget, -}; - -pub struct Panel<'a, Message, Renderer> { - style: Style, - content: Box<Widget<Message, Renderer> + 'a>, -} - -impl<'a, Message, Renderer> Panel<'a, Message, Renderer> { - pub fn new(content: impl Widget<Message, Renderer> + 'a) -> Self { - Panel { - style: Style::default().padding(20), - content: Box::new(content), - } - } - - pub fn width(mut self, width: u32) -> Self { - self.style = self.style.width(width); - self - } - - pub fn max_width(mut self, max_width: u32) -> Self { - self.style = self.style.max_width(max_width); - self - } -} - -impl<'a, Message, Renderer> Widget<Message, Renderer> - for Panel<'a, Message, Renderer> -where - Renderer: self::Renderer, -{ - fn node(&self, renderer: &Renderer) -> Node { - Node::with_children(self.style, vec![self.content.node(renderer)]) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - [&mut self.content] - .iter_mut() - .zip(layout.children()) - .for_each(|(child, layout)| { - child.on_event(event, layout, cursor_position, messages) - }); - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout, - cursor_position: Point, - ) -> MouseCursor { - let bounds = layout.bounds(); - let mut cursor = MouseCursor::OutOfBounds; - renderer.draw(bounds); - - [&self.content].iter().zip(layout.children()).for_each( - |(child, layout)| { - let new_cursor = child.draw(renderer, layout, cursor_position); - - if new_cursor != MouseCursor::OutOfBounds { - cursor = new_cursor; - } - }, - ); - - if cursor == MouseCursor::OutOfBounds { - if bounds.contains(cursor_position) { - MouseCursor::Idle - } else { - MouseCursor::OutOfBounds - } - } else { - cursor - } - } - - fn hash(&self, state: &mut Hasher) { - self.style.hash(state); - } -} - -pub trait Renderer { - fn draw(&mut self, bounds: Rectangle); -} diff --git a/src/widget/progress_bar.rs b/src/widget/progress_bar.rs deleted file mode 100644 index d4499160..00000000 --- a/src/widget/progress_bar.rs +++ /dev/null @@ -1,106 +0,0 @@ -//! Provide visual feedback to your users when performing a slow task. - -use crate::{ - Element, Hasher, Layout, MouseCursor, Node, Point, Rectangle, Style, Widget, -}; - -use std::hash::Hash; - -/// A bar that is filled based on an amount of progress. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`progress_bar::Renderer`] trait. -/// -/// [`Widget`]: ../trait.Widget.html -/// [`progress_bar::Renderer`]: trait.Renderer.html -/// -/// # Example -/// -/// ``` -/// use iced::ProgressBar; -/// -/// let progress = 0.75; -/// -/// ProgressBar::new(progress); -/// ``` -#[derive(Debug)] -pub struct ProgressBar { - progress: f32, - style: Style, -} - -impl ProgressBar { - /// Creates a new [`ProgressBar`] filled based on the given amount of - /// progress. - /// - /// The progress should be in the `0.0..=1.0` range. `0` meaning no work - /// done, and `1` meaning work finished. - /// - /// [`ProgressBar`]: struct.ProgressBar.html - pub fn new(progress: f32) -> Self { - ProgressBar { - progress, - style: Style::default().fill_width(), - } - } - - /// Sets the width of the [`ProgressBar`] in pixels. - /// - /// [`ProgressBar`]: struct.ProgressBar.html - pub fn width(mut self, width: u16) -> Self { - self.style = self.style.width(width); - self - } -} - -impl<Message, Renderer> Widget<Message, Renderer> for ProgressBar -where - Renderer: self::Renderer, -{ - fn node(&self, _renderer: &Renderer) -> Node { - Node::new(self.style.height(50)) - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - _cursor_position: Point, - ) -> MouseCursor { - renderer.draw(layout.bounds(), self.progress); - - MouseCursor::OutOfBounds - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - } -} - -/// The renderer of a [`ProgressBar`]. -/// -/// Your [renderer] will need to implement this trait before being able to use -/// a [`ProgressBar`] in your user interface. -/// -/// [`ProgressBar`]: struct.ProgressBar.html -/// [renderer]: ../../renderer/index.html -pub trait Renderer { - /// Draws a [`ProgressBar`]. - /// - /// It receives: - /// * the bounds of the [`ProgressBar`] - /// * the current progress of the [`ProgressBar`], in the `0.0..=1.0` - /// range. - /// - /// [`ProgressBar`]: struct.ProgressBar.html - fn draw(&mut self, bounds: Rectangle, progress: f32); -} - -impl<'a, Message, Renderer> From<ProgressBar> for Element<'a, Message, Renderer> -where - Renderer: self::Renderer, -{ - fn from(progress_bar: ProgressBar) -> Element<'a, Message, Renderer> { - Element::new(progress_bar) - } -} diff --git a/src/widget/radio.rs b/src/widget/radio.rs deleted file mode 100644 index 048aea94..00000000 --- a/src/widget/radio.rs +++ /dev/null @@ -1,207 +0,0 @@ -//! Create choices using radio buttons. -use crate::input::{mouse, ButtonState}; -use crate::widget::{text, Column, Row, Text}; -use crate::{ - Align, Color, Element, Event, Hasher, Layout, MouseCursor, Node, Point, - Rectangle, Widget, -}; - -use std::hash::Hash; - -/// A circular button representing a choice. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`radio::Renderer`] trait. -/// -/// [`Widget`]: ../trait.Widget.html -/// [`radio::Renderer`]: trait.Renderer.html -/// -/// # Example -/// ``` -/// use iced::Radio; -/// -/// #[derive(Debug, Clone, Copy, PartialEq, Eq)] -/// pub enum Choice { -/// A, -/// B, -/// } -/// -/// #[derive(Debug, Clone, Copy)] -/// pub enum Message { -/// RadioSelected(Choice), -/// } -/// -/// let selected_choice = Some(Choice::A); -/// -/// Radio::new(Choice::A, "This is A", selected_choice, Message::RadioSelected); -/// -/// Radio::new(Choice::B, "This is B", selected_choice, Message::RadioSelected); -/// ``` -/// -/// 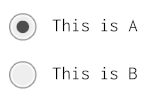 -pub struct Radio<Message> { - /// Whether the radio button is selected or not - pub is_selected: bool, - - /// The message to produce when the radio button is clicked - pub on_click: Message, - - /// The label of the radio button - pub label: String, - - /// The color of the label - pub label_color: Option<Color>, -} - -impl<Message> std::fmt::Debug for Radio<Message> -where - Message: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Radio") - .field("is_selected", &self.is_selected) - .field("on_click", &self.on_click) - .field("label", &self.label) - .field("label_color", &self.label_color) - .finish() - } -} - -impl<Message> Radio<Message> { - /// Creates a new [`Radio`] button. - /// - /// It expects: - /// * the value related to the [`Radio`] button - /// * the label of the [`Radio`] button - /// * the current selected value - /// * a function that will be called when the [`Radio`] is selected. It - /// receives the value of the radio and must produce a `Message`. - /// - /// [`Radio`]: struct.Radio.html - pub fn new<F, V>(value: V, label: &str, selected: Option<V>, f: F) -> Self - where - V: Eq + Copy, - F: 'static + Fn(V) -> Message, - { - Radio { - is_selected: Some(value) == selected, - on_click: f(value), - label: String::from(label), - label_color: None, - } - } - - /// Sets the `Color` of the label of the [`Radio`]. - /// - /// [`Radio`]: struct.Radio.html - pub fn label_color<C: Into<Color>>(mut self, color: C) -> Self { - self.label_color = Some(color.into()); - self - } -} - -impl<Message, Renderer> Widget<Message, Renderer> for Radio<Message> -where - Renderer: self::Renderer + text::Renderer, - Message: Copy + std::fmt::Debug, -{ - fn node(&self, renderer: &mut Renderer) -> Node { - Row::<(), Renderer>::new() - .spacing(15) - .align_items(Align::Center) - .push(Column::new().width(28).height(28)) - .push(Text::new(&self.label)) - .node(renderer) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - match event { - Event::Mouse(mouse::Event::Input { - button: mouse::Button::Left, - state: ButtonState::Pressed, - }) => { - if layout.bounds().contains(cursor_position) { - messages.push(self.on_click); - } - } - _ => {} - } - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - cursor_position: Point, - ) -> MouseCursor { - let children: Vec<_> = layout.children().collect(); - - let mut text_bounds = children[1].bounds(); - text_bounds.y -= 2.0; - - text::Renderer::draw( - renderer, - text_bounds, - &self.label, - None, - self.label_color, - text::HorizontalAlignment::Left, - text::VerticalAlignment::Top, - ); - - self::Renderer::draw( - renderer, - cursor_position, - children[0].bounds(), - layout.bounds(), - self.is_selected, - ) - } - - fn hash_layout(&self, state: &mut Hasher) { - self.label.hash(state); - } -} - -/// The renderer of a [`Radio`] button. -/// -/// Your [renderer] will need to implement this trait before being -/// able to use a [`Radio`] button in your user interface. -/// -/// [`Radio`]: struct.Radio.html -/// [renderer]: ../../renderer/index.html -pub trait Renderer { - /// Draws a [`Radio`] button. - /// - /// It receives: - /// * the current cursor position - /// * the bounds of the [`Radio`] - /// * the bounds of the label of the [`Radio`] - /// * whether the [`Radio`] is selected or not - /// - /// [`Radio`]: struct.Radio.html - fn draw( - &mut self, - cursor_position: Point, - bounds: Rectangle, - label_bounds: Rectangle, - is_selected: bool, - ) -> MouseCursor; -} - -impl<'a, Message, Renderer> From<Radio<Message>> - for Element<'a, Message, Renderer> -where - Renderer: self::Renderer + text::Renderer, - Message: 'static + Copy + std::fmt::Debug, -{ - fn from(checkbox: Radio<Message>) -> Element<'a, Message, Renderer> { - Element::new(checkbox) - } -} diff --git a/src/widget/row.rs b/src/widget/row.rs deleted file mode 100644 index 181020e3..00000000 --- a/src/widget/row.rs +++ /dev/null @@ -1,219 +0,0 @@ -use std::hash::Hash; - -use crate::{ - Align, Element, Event, Hasher, Justify, Layout, MouseCursor, Node, Point, - Style, Widget, -}; - -/// A container that distributes its contents horizontally. -/// -/// A [`Row`] will try to fill the horizontal space of its container. -/// -/// [`Row`]: struct.Row.html -#[derive(Default)] -pub struct Row<'a, Message, Renderer> { - style: Style, - spacing: u16, - children: Vec<Element<'a, Message, Renderer>>, -} - -impl<'a, Message, Renderer> std::fmt::Debug for Row<'a, Message, Renderer> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Row") - .field("style", &self.style) - .field("spacing", &self.spacing) - .field("children", &self.children) - .finish() - } -} - -impl<'a, Message, Renderer> Row<'a, Message, Renderer> { - /// Creates an empty [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn new() -> Self { - Row { - style: Style::default().fill_width(), - spacing: 0, - children: Vec::new(), - } - } - - /// Sets the horizontal spacing _between_ elements in pixels. - /// - /// Custom margins per element do not exist in Iced. You should use this - /// method instead! While less flexible, it helps you keep spacing between - /// elements consistent. - pub fn spacing(mut self, px: u16) -> Self { - self.spacing = px; - self - } - - /// Sets the padding of the [`Row`] in pixels. - /// - /// [`Row`]: struct.Row.html - pub fn padding(mut self, px: u16) -> Self { - self.style = self.style.padding(px); - self - } - - /// Sets the width of the [`Row`] in pixels. - /// - /// [`Row`]: struct.Row.html - pub fn width(mut self, width: u16) -> Self { - self.style = self.style.width(width); - self - } - - /// Sets the height of the [`Row`] in pixels. - /// - /// [`Row`]: struct.Row.html - pub fn height(mut self, height: u16) -> Self { - self.style = self.style.height(height); - self - } - - /// Sets the maximum width of the [`Row`] in pixels. - /// - /// [`Row`]: struct.Row.html - pub fn max_width(mut self, max_width: u16) -> Self { - self.style = self.style.max_width(max_width); - self - } - - /// Sets the maximum height of the [`Row`] in pixels. - /// - /// [`Row`]: struct.Row.html - pub fn max_height(mut self, max_height: u16) -> Self { - self.style = self.style.max_height(max_height); - self - } - - /// Sets the alignment of the [`Row`] itself. - /// - /// This is useful if you want to override the default alignment given by - /// the parent container. - /// - /// [`Row`]: struct.Row.html - pub fn align_self(mut self, align: Align) -> Self { - self.style = self.style.align_self(align); - self - } - - /// Sets the vertical alignment of the contents of the [`Row`] . - /// - /// [`Row`]: struct.Row.html - pub fn align_items(mut self, align: Align) -> Self { - self.style = self.style.align_items(align); - self - } - - /// Sets the horizontal distribution strategy for the contents of the - /// [`Row`] . - /// - /// [`Row`]: struct.Row.html - pub fn justify_content(mut self, justify: Justify) -> Self { - self.style = self.style.justify_content(justify); - self - } - - /// Adds an [`Element`] to the [`Row`]. - /// - /// [`Element`]: ../struct.Element.html - /// [`Row`]: struct.Row.html - pub fn push<E>(mut self, child: E) -> Row<'a, Message, Renderer> - where - E: Into<Element<'a, Message, Renderer>>, - { - self.children.push(child.into()); - self - } -} - -impl<'a, Message, Renderer> Widget<Message, Renderer> - for Row<'a, Message, Renderer> -{ - fn node(&self, renderer: &mut Renderer) -> Node { - let mut children: Vec<Node> = self - .children - .iter() - .map(|child| { - let mut node = child.widget.node(renderer); - - let mut style = node.0.style(); - style.margin.end = - stretch::style::Dimension::Points(f32::from(self.spacing)); - - node.0.set_style(style); - node - }) - .collect(); - - if let Some(node) = children.last_mut() { - let mut style = node.0.style(); - style.margin.end = stretch::style::Dimension::Undefined; - - node.0.set_style(style); - } - - Node::with_children(self.style, children) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - self.children.iter_mut().zip(layout.children()).for_each( - |(child, layout)| { - child - .widget - .on_event(event, layout, cursor_position, messages) - }, - ); - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - cursor_position: Point, - ) -> MouseCursor { - let mut cursor = MouseCursor::OutOfBounds; - - self.children.iter().zip(layout.children()).for_each( - |(child, layout)| { - let new_cursor = - child.widget.draw(renderer, layout, cursor_position); - - if new_cursor != MouseCursor::OutOfBounds { - cursor = new_cursor; - } - }, - ); - - cursor - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - self.spacing.hash(state); - - for child in &self.children { - child.widget.hash_layout(state); - } - } -} - -impl<'a, Message, Renderer> From<Row<'a, Message, Renderer>> - for Element<'a, Message, Renderer> -where - Renderer: 'a, - Message: 'static, -{ - fn from(row: Row<'a, Message, Renderer>) -> Element<'a, Message, Renderer> { - Element::new(row) - } -} diff --git a/src/widget/slider.rs b/src/widget/slider.rs deleted file mode 100644 index fb6db8c9..00000000 --- a/src/widget/slider.rs +++ /dev/null @@ -1,245 +0,0 @@ -//! Display an interactive selector of a single value from a range of values. -//! -//! A [`Slider`] has some local [`State`]. -//! -//! [`Slider`]: struct.Slider.html -//! [`State`]: struct.State.html -use std::hash::Hash; -use std::ops::RangeInclusive; -use std::rc::Rc; - -use crate::input::{mouse, ButtonState}; -use crate::{ - Element, Event, Hasher, Layout, MouseCursor, Node, Point, Rectangle, Style, - Widget, -}; - -/// An horizontal bar and a handle that selects a single value from a range of -/// values. -/// -/// A [`Slider`] will try to fill the horizontal space of its container. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`slider::Renderer`] trait. -/// -/// [`Slider`]: struct.Slider.html -/// [`Widget`]: ../trait.Widget.html -/// [`slider::Renderer`]: trait.Renderer.html -/// -/// # Example -/// ``` -/// use iced::{slider, Slider}; -/// -/// pub enum Message { -/// SliderChanged(f32), -/// } -/// -/// let state = &mut slider::State::new(); -/// let value = 50.0; -/// -/// Slider::new(state, 0.0..=100.0, value, Message::SliderChanged); -/// ``` -/// -///  -pub struct Slider<'a, Message> { - state: &'a mut State, - /// The range of the slider - pub range: RangeInclusive<f32>, - /// The current value of the slider - pub value: f32, - /// The function to produce messages on change - pub on_change: Rc<Box<dyn Fn(f32) -> Message>>, - style: Style, -} - -impl<'a, Message> std::fmt::Debug for Slider<'a, Message> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Slider") - .field("state", &self.state) - .field("range", &self.range) - .field("value", &self.value) - .field("style", &self.style) - .finish() - } -} - -impl<'a, Message> Slider<'a, Message> { - /// Creates a new [`Slider`]. - /// - /// It expects: - /// * the local [`State`] of the [`Slider`] - /// * an inclusive range of possible values - /// * the current value of the [`Slider`] - /// * a function that will be called when the [`Slider`] is dragged. - /// It receives the new value of the [`Slider`] and must produce a - /// `Message`. - /// - /// [`Slider`]: struct.Slider.html - /// [`State`]: struct.State.html - pub fn new<F>( - state: &'a mut State, - range: RangeInclusive<f32>, - value: f32, - on_change: F, - ) -> Self - where - F: 'static + Fn(f32) -> Message, - { - Slider { - state, - value: value.max(*range.start()).min(*range.end()), - range, - on_change: Rc::new(Box::new(on_change)), - style: Style::default().min_width(100).fill_width(), - } - } - - /// Sets the width of the [`Slider`] in pixels. - /// - /// [`Slider`]: struct.Slider.html - pub fn width(mut self, width: u16) -> Self { - self.style = self.style.width(width); - self - } -} - -impl<'a, Message, Renderer> Widget<Message, Renderer> for Slider<'a, Message> -where - Renderer: self::Renderer, -{ - fn node(&self, _renderer: &mut Renderer) -> Node { - Node::new(self.style.height(25)) - } - - fn on_event( - &mut self, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - messages: &mut Vec<Message>, - ) { - let mut change = || { - let bounds = layout.bounds(); - - if cursor_position.x <= bounds.x { - messages.push((self.on_change)(*self.range.start())); - } else if cursor_position.x >= bounds.x + bounds.width { - messages.push((self.on_change)(*self.range.end())); - } else { - let percent = (cursor_position.x - bounds.x) / bounds.width; - let value = (self.range.end() - self.range.start()) * percent - + self.range.start(); - - messages.push((self.on_change)(value)); - } - }; - - match event { - Event::Mouse(mouse::Event::Input { - button: mouse::Button::Left, - state, - }) => match state { - ButtonState::Pressed => { - if layout.bounds().contains(cursor_position) { - change(); - self.state.is_dragging = true; - } - } - ButtonState::Released => { - self.state.is_dragging = false; - } - }, - Event::Mouse(mouse::Event::CursorMoved { .. }) => { - if self.state.is_dragging { - change(); - } - } - _ => {} - } - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - cursor_position: Point, - ) -> MouseCursor { - renderer.draw( - cursor_position, - layout.bounds(), - self.state, - self.range.clone(), - self.value, - ) - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - } -} - -/// The local state of a [`Slider`]. -/// -/// [`Slider`]: struct.Slider.html -#[derive(Debug, Clone, Copy, PartialEq, Eq, Default)] -pub struct State { - is_dragging: bool, -} - -impl State { - /// Creates a new [`State`]. - /// - /// [`State`]: struct.State.html - pub fn new() -> State { - State::default() - } - - /// Returns whether the associated [`Slider`] is currently being dragged or - /// not. - /// - /// [`Slider`]: struct.Slider.html - pub fn is_dragging(&self) -> bool { - self.is_dragging - } -} - -/// The renderer of a [`Slider`]. -/// -/// Your [renderer] will need to implement this trait before being -/// able to use a [`Slider`] in your user interface. -/// -/// [`Slider`]: struct.Slider.html -/// [renderer]: ../../renderer/index.html -pub trait Renderer { - /// Draws a [`Slider`]. - /// - /// It receives: - /// * the current cursor position - /// * the bounds of the [`Slider`] - /// * the local state of the [`Slider`] - /// * the range of values of the [`Slider`] - /// * the current value of the [`Slider`] - /// - /// [`Slider`]: struct.Slider.html - /// [`State`]: struct.State.html - /// [`Class`]: enum.Class.html - fn draw( - &mut self, - cursor_position: Point, - bounds: Rectangle, - state: &State, - range: RangeInclusive<f32>, - value: f32, - ) -> MouseCursor; -} - -impl<'a, Message, Renderer> From<Slider<'a, Message>> - for Element<'a, Message, Renderer> -where - Renderer: self::Renderer, - Message: 'static, -{ - fn from(slider: Slider<'a, Message>) -> Element<'a, Message, Renderer> { - Element::new(slider) - } -} diff --git a/src/widget/text.rs b/src/widget/text.rs deleted file mode 100644 index 4ef10d52..00000000 --- a/src/widget/text.rs +++ /dev/null @@ -1,218 +0,0 @@ -//! Write some text for your users to read. -use crate::{ - Color, Element, Hasher, Layout, MouseCursor, Node, Point, Rectangle, Style, - Widget, -}; - -use std::hash::Hash; - -/// A paragraph of text. -/// -/// It implements [`Widget`] when the associated `Renderer` implements the -/// [`text::Renderer`] trait. -/// -/// [`Widget`]: ../trait.Widget.html -/// [`text::Renderer`]: trait.Renderer.html -/// -/// # Example -/// -/// ``` -/// use iced::{Text, Color}; -/// -/// Text::new("I <3 iced!") -/// .size(40); -/// ``` -#[derive(Debug, Clone)] -pub struct Text { - /// The text contents - pub content: String, - /// The text size - pub size: Option<u16>, - color: Option<Color>, - style: Style, - horizontal_alignment: HorizontalAlignment, - vertical_alignment: VerticalAlignment, -} - -impl Text { - /// Create a new fragment of [`Text`] with the given contents. - /// - /// [`Text`]: struct.Text.html - pub fn new(label: &str) -> Self { - Text { - content: String::from(label), - size: None, - color: None, - style: Style::default().fill_width(), - horizontal_alignment: HorizontalAlignment::Left, - vertical_alignment: VerticalAlignment::Top, - } - } - - /// Sets the size of the [`Text`] in pixels. - /// - /// [`Text`]: struct.Text.html - pub fn size(mut self, size: u16) -> Self { - self.size = Some(size); - self - } - - /// Sets the `Color` of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - pub fn color<C: Into<Color>>(mut self, color: C) -> Self { - self.color = Some(color.into()); - self - } - - /// Sets the width of the [`Text`] boundaries in pixels. - /// - /// [`Text`]: struct.Text.html - pub fn width(mut self, width: u16) -> Self { - self.style = self.style.width(width); - self - } - - /// Sets the height of the [`Text`] boundaries in pixels. - /// - /// [`Text`]: struct.Text.html - pub fn height(mut self, height: u16) -> Self { - self.style = self.style.height(height); - self - } - - /// Sets the [`HorizontalAlignment`] of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - /// [`HorizontalAlignment`]: enum.HorizontalAlignment.html - pub fn horizontal_alignment( - mut self, - alignment: HorizontalAlignment, - ) -> Self { - self.horizontal_alignment = alignment; - self - } - - /// Sets the [`VerticalAlignment`] of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - /// [`VerticalAlignment`]: enum.VerticalAlignment.html - pub fn vertical_alignment(mut self, alignment: VerticalAlignment) -> Self { - self.vertical_alignment = alignment; - self - } -} - -impl<Message, Renderer> Widget<Message, Renderer> for Text -where - Renderer: self::Renderer, -{ - fn node(&self, renderer: &mut Renderer) -> Node { - renderer.node(self.style, &self.content, self.size) - } - - fn draw( - &self, - renderer: &mut Renderer, - layout: Layout<'_>, - _cursor_position: Point, - ) -> MouseCursor { - renderer.draw( - layout.bounds(), - &self.content, - self.size, - self.color, - self.horizontal_alignment, - self.vertical_alignment, - ); - - MouseCursor::OutOfBounds - } - - fn hash_layout(&self, state: &mut Hasher) { - self.style.hash(state); - - self.content.hash(state); - self.size.hash(state); - } -} - -/// The renderer of a [`Text`] fragment. -/// -/// Your [renderer] will need to implement this trait before being -/// able to use [`Text`] in your [`UserInterface`]. -/// -/// [`Text`]: struct.Text.html -/// [renderer]: ../../renderer/index.html -/// [`UserInterface`]: ../../struct.UserInterface.html -pub trait Renderer { - /// Creates a [`Node`] with the given [`Style`] for the provided [`Text`] - /// contents and size. - /// - /// You should probably use [`Node::with_measure`] to allow [`Text`] to - /// adapt to the dimensions of its container. - /// - /// [`Node`]: ../../struct.Node.html - /// [`Style`]: ../../struct.Style.html - /// [`Text`]: struct.Text.html - /// [`Node::with_measure`]: ../../struct.Node.html#method.with_measure - fn node(&self, style: Style, content: &str, size: Option<u16>) -> Node; - - /// Draws a [`Text`] fragment. - /// - /// It receives: - /// * the bounds of the [`Text`] - /// * the contents of the [`Text`] - /// * the size of the [`Text`] - /// * the color of the [`Text`] - /// * the [`HorizontalAlignment`] of the [`Text`] - /// * the [`VerticalAlignment`] of the [`Text`] - /// - /// [`Text`]: struct.Text.html - /// [`HorizontalAlignment`]: enum.HorizontalAlignment.html - /// [`VerticalAlignment`]: enum.VerticalAlignment.html - fn draw( - &mut self, - bounds: Rectangle, - content: &str, - size: Option<u16>, - color: Option<Color>, - horizontal_alignment: HorizontalAlignment, - vertical_alignment: VerticalAlignment, - ); -} - -impl<'a, Message, Renderer> From<Text> for Element<'a, Message, Renderer> -where - Renderer: self::Renderer, -{ - fn from(text: Text) -> Element<'a, Message, Renderer> { - Element::new(text) - } -} - -/// The horizontal alignment of some resource. -#[derive(Debug, Clone, Copy, PartialEq, Eq)] -pub enum HorizontalAlignment { - /// Align left - Left, - - /// Horizontally centered - Center, - - /// Align right - Right, -} - -/// The vertical alignment of some resource. -#[derive(Debug, Clone, Copy, PartialEq, Eq)] -pub enum VerticalAlignment { - /// Align top - Top, - - /// Vertically centered - Center, - - /// Align bottom - Bottom, -} |