diff options
Diffstat (limited to 'src/widget')
-rw-r--r-- | src/widget/button.rs | 19 | ||||
-rw-r--r-- | src/widget/checkbox.rs | 52 | ||||
-rw-r--r-- | src/widget/column.rs | 13 | ||||
-rw-r--r-- | src/widget/panel.rs | 2 | ||||
-rw-r--r-- | src/widget/radio.rs | 48 | ||||
-rw-r--r-- | src/widget/row.rs | 13 | ||||
-rw-r--r-- | src/widget/slider.rs | 17 | ||||
-rw-r--r-- | src/widget/text.rs | 65 |
8 files changed, 108 insertions, 121 deletions
diff --git a/src/widget/button.rs b/src/widget/button.rs index 35fa6ca8..14fd3852 100644 --- a/src/widget/button.rs +++ b/src/widget/button.rs @@ -16,11 +16,10 @@ use std::hash::Hash; /// A generic widget that produces a message when clicked. /// -/// It implements [`Widget`] when the associated [`core::Renderer`] implements -/// the [`button::Renderer`] trait. +/// It implements [`Widget`] when the associated `Renderer` implements the +/// [`button::Renderer`] trait. /// -/// [`Widget`]: ../../core/trait.Widget.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [`Widget`]: ../trait.Widget.html /// [`button::Renderer`]: trait.Renderer.html /// /// # Example @@ -37,6 +36,8 @@ use std::hash::Hash; /// Button::new(state, "Click me!") /// .on_press(Message::ButtonClicked); /// ``` +/// +///  pub struct Button<'a, Message> { state: &'a mut State, label: String, @@ -186,7 +187,7 @@ where ) } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.style.hash(state); } } @@ -218,7 +219,7 @@ impl State { /// The type of a [`Button`]. /// -/// 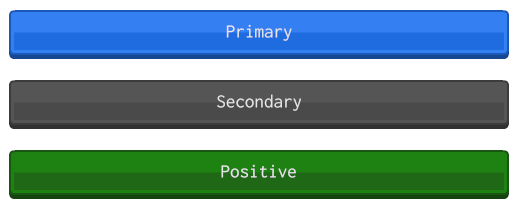 +/// 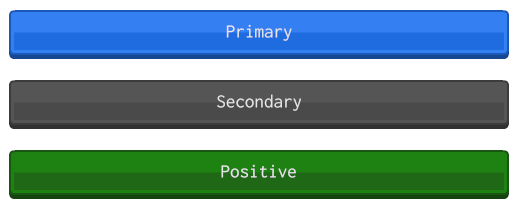 /// /// [`Button`]: struct.Button.html #[derive(Debug, Clone, Copy, PartialEq, Eq)] @@ -241,11 +242,11 @@ pub enum Class { /// The renderer of a [`Button`]. /// -/// Your [`core::Renderer`] will need to implement this trait before being +/// Your [renderer] will need to implement this trait before being /// able to use a [`Button`] in your user interface. /// /// [`Button`]: struct.Button.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [renderer]: ../../renderer/index.html pub trait Renderer { /// Draws a [`Button`]. /// @@ -262,7 +263,7 @@ pub trait Renderer { fn draw( &mut self, cursor_position: Point, - bounds: Rectangle<f32>, + bounds: Rectangle, state: &State, label: &str, class: Class, diff --git a/src/widget/checkbox.rs b/src/widget/checkbox.rs index c30b8308..b9a40a37 100644 --- a/src/widget/checkbox.rs +++ b/src/widget/checkbox.rs @@ -8,13 +8,12 @@ use crate::{ Widget, }; -/// A box that can be checked. +/// A box that can be checked, with a generic text `Color`. /// -/// It implements [`Widget`] when the [`core::Renderer`] implements the +/// It implements [`Widget`] when the associated `Renderer` implements the /// [`checkbox::Renderer`] trait. /// -/// [`Widget`]: ../../core/trait.Widget.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [`Widget`]: ../trait.Widget.html /// [`checkbox::Renderer`]: trait.Renderer.html /// /// # Example @@ -25,29 +24,24 @@ use crate::{ /// #[derive(Debug, Clone, Copy)] /// pub enum Color { /// Black, -/// White, -/// } -/// -/// impl Default for Color { -/// fn default() -> Color { -/// Color::Black -/// } /// } /// /// pub enum Message { /// CheckboxToggled(bool), /// } /// -/// fn some_checkbox(is_checked: bool) -> Checkbox<Color, Message> { -/// Checkbox::new(is_checked, "Toggle me!", Message::CheckboxToggled) -/// .label_color(Color::White) -/// } +/// let is_checked = true; +/// +/// Checkbox::new(is_checked, "Toggle me!", Message::CheckboxToggled) +/// .label_color(Color::Black); /// ``` +/// +/// 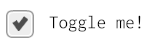 pub struct Checkbox<Color, Message> { is_checked: bool, on_toggle: Box<dyn Fn(bool) -> Message>, label: String, - label_color: Color, + label_color: Option<Color>, } impl<Color, Message> std::fmt::Debug for Checkbox<Color, Message> @@ -63,10 +57,7 @@ where } } -impl<Color, Message> Checkbox<Color, Message> -where - Color: Default, -{ +impl<Color, Message> Checkbox<Color, Message> { /// Creates a new [`Checkbox`]. /// /// It expects: @@ -85,16 +76,15 @@ where is_checked, on_toggle: Box::new(f), label: String::from(label), - label_color: Color::default(), + label_color: None, } } - /// Sets the [`Color`] of the label of the [`Checkbox`]. + /// Sets the `Color` of the label of the [`Checkbox`]. /// - /// [`Color`]: ../../../../graphics/struct.Color.html /// [`Checkbox`]: struct.Checkbox.html pub fn label_color(mut self, color: Color) -> Self { - self.label_color = color; + self.label_color = Some(color); self } } @@ -102,7 +92,7 @@ where impl<Color, Message, Renderer> Widget<Message, Renderer> for Checkbox<Color, Message> where - Color: 'static + Copy + Default + std::fmt::Debug, + Color: 'static + Copy + std::fmt::Debug, Renderer: self::Renderer + text::Renderer<Color>, { fn node(&self, renderer: &Renderer) -> Node { @@ -167,18 +157,18 @@ where ) } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.label.hash(state); } } /// The renderer of a [`Checkbox`]. /// -/// Your [`core::Renderer`] will need to implement this trait before being +/// Your [renderer] will need to implement this trait before being /// able to use a [`Checkbox`] in your user interface. /// /// [`Checkbox`]: struct.Checkbox.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [renderer]: ../../renderer/index.html pub trait Renderer { /// Draws a [`Checkbox`]. /// @@ -192,8 +182,8 @@ pub trait Renderer { fn draw( &mut self, cursor_position: Point, - bounds: Rectangle<f32>, - label_bounds: Rectangle<f32>, + bounds: Rectangle, + label_bounds: Rectangle, is_checked: bool, ) -> MouseCursor; } @@ -201,7 +191,7 @@ pub trait Renderer { impl<'a, Color, Message, Renderer> From<Checkbox<Color, Message>> for Element<'a, Message, Renderer> where - Color: 'static + Copy + Default + std::fmt::Debug, + Color: 'static + Copy + std::fmt::Debug, Renderer: self::Renderer + text::Renderer<Color>, Message: 'static, { diff --git a/src/widget/column.rs b/src/widget/column.rs index 18a68f60..903de897 100644 --- a/src/widget/column.rs +++ b/src/widget/column.rs @@ -5,11 +5,12 @@ use crate::{ Style, Widget, }; -/// A container that places its contents vertically. +/// A container that distributes its contents vertically. /// /// A [`Column`] will try to fill the horizontal space of its container. /// /// [`Column`]: struct.Column.html +#[derive(Default)] pub struct Column<'a, Message, Renderer> { style: Style, spacing: u16, @@ -43,7 +44,7 @@ impl<'a, Message, Renderer> Column<'a, Message, Renderer> { /// Sets the vertical spacing _between_ elements in pixels. /// - /// Custom margins per element do not exist in Coffee. You should use this + /// Custom margins per element do not exist in Iced. You should use this /// method instead! While less flexible, it helps you keep spacing between /// elements consistent. pub fn spacing(mut self, px: u16) -> Self { @@ -121,7 +122,7 @@ impl<'a, Message, Renderer> Column<'a, Message, Renderer> { /// Adds an [`Element`] to the [`Column`]. /// - /// [`Element`]: ../core/struct.Element.html + /// [`Element`]: ../struct.Element.html /// [`Column`]: struct.Column.html pub fn push<E>(mut self, child: E) -> Column<'a, Message, Renderer> where @@ -144,7 +145,7 @@ impl<'a, Message, Renderer> Widget<Message, Renderer> let mut style = node.0.style(); style.margin.bottom = - stretch::style::Dimension::Points(self.spacing as f32); + stretch::style::Dimension::Points(f32::from(self.spacing)); node.0.set_style(style); node @@ -199,12 +200,12 @@ impl<'a, Message, Renderer> Widget<Message, Renderer> cursor } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.style.hash(state); self.spacing.hash(state); for child in &self.children { - child.widget.hash(state); + child.widget.hash_layout(state); } } } diff --git a/src/widget/panel.rs b/src/widget/panel.rs index 28e5989c..d43d6fb6 100644 --- a/src/widget/panel.rs +++ b/src/widget/panel.rs @@ -90,5 +90,5 @@ where } pub trait Renderer { - fn draw(&mut self, bounds: Rectangle<f32>); + fn draw(&mut self, bounds: Rectangle); } diff --git a/src/widget/radio.rs b/src/widget/radio.rs index 8a678ec8..a59d52aa 100644 --- a/src/widget/radio.rs +++ b/src/widget/radio.rs @@ -8,13 +8,12 @@ use crate::{ use std::hash::Hash; -/// A circular button representing a choice. +/// A circular button representing a choice, with a generic text `Color`. /// -/// It implements [`Widget`] when the [`core::Renderer`] implements the +/// It implements [`Widget`] when the associated `Renderer` implements the /// [`radio::Renderer`] trait. /// -/// [`Widget`]: ../../core/trait.Widget.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [`Widget`]: ../trait.Widget.html /// [`radio::Renderer`]: trait.Renderer.html /// /// # Example @@ -26,12 +25,6 @@ use std::hash::Hash; /// Black, /// } /// -/// impl Default for Color { -/// fn default() -> Color { -/// Color::Black -/// } -/// } -/// /// #[derive(Debug, Clone, Copy, PartialEq, Eq)] /// pub enum Choice { /// A, @@ -45,16 +38,19 @@ use std::hash::Hash; /// /// let selected_choice = Some(Choice::A); /// -/// Radio::<Color, Message>::new(Choice::A, "This is A", selected_choice, Message::RadioSelected) +/// Radio::new(Choice::A, "This is A", selected_choice, Message::RadioSelected) +/// .label_color(Color::Black); +/// +/// Radio::new(Choice::B, "This is B", selected_choice, Message::RadioSelected) /// .label_color(Color::Black); /// ``` /// -/// 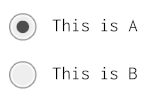 +/// 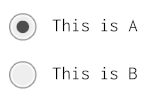 pub struct Radio<Color, Message> { is_selected: bool, on_click: Message, label: String, - label_color: Color, + label_color: Option<Color>, } impl<Color, Message> std::fmt::Debug for Radio<Color, Message> @@ -72,10 +68,7 @@ where } } -impl<Color, Message> Radio<Color, Message> -where - Color: Default, -{ +impl<Color, Message> Radio<Color, Message> { /// Creates a new [`Radio`] button. /// /// It expects: @@ -95,16 +88,15 @@ where is_selected: Some(value) == selected, on_click: f(value), label: String::from(label), - label_color: Color::default(), + label_color: None, } } - /// Sets the [`Color`] of the label of the [`Radio`]. + /// Sets the `Color` of the label of the [`Radio`]. /// - /// [`Color`]: ../../../../graphics/struct.Color.html /// [`Radio`]: struct.Radio.html pub fn label_color(mut self, color: Color) -> Self { - self.label_color = color; + self.label_color = Some(color); self } } @@ -112,7 +104,7 @@ where impl<Color, Message, Renderer> Widget<Message, Renderer> for Radio<Color, Message> where - Color: 'static + Copy + Default + std::fmt::Debug, + Color: 'static + Copy + std::fmt::Debug, Renderer: self::Renderer + text::Renderer<Color>, Message: Copy + std::fmt::Debug, { @@ -175,18 +167,18 @@ where ) } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.label.hash(state); } } /// The renderer of a [`Radio`] button. /// -/// Your [`core::Renderer`] will need to implement this trait before being +/// Your [renderer] will need to implement this trait before being /// able to use a [`Radio`] button in your user interface. /// /// [`Radio`]: struct.Radio.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [renderer]: ../../renderer/index.html pub trait Renderer { /// Draws a [`Radio`] button. /// @@ -200,8 +192,8 @@ pub trait Renderer { fn draw( &mut self, cursor_position: Point, - bounds: Rectangle<f32>, - label_bounds: Rectangle<f32>, + bounds: Rectangle, + label_bounds: Rectangle, is_selected: bool, ) -> MouseCursor; } @@ -209,7 +201,7 @@ pub trait Renderer { impl<'a, Color, Message, Renderer> From<Radio<Color, Message>> for Element<'a, Message, Renderer> where - Color: 'static + Copy + Default + std::fmt::Debug, + Color: 'static + Copy + std::fmt::Debug, Renderer: self::Renderer + text::Renderer<Color>, Message: 'static + Copy + std::fmt::Debug, { diff --git a/src/widget/row.rs b/src/widget/row.rs index 9e0f9d4c..7b7033a1 100644 --- a/src/widget/row.rs +++ b/src/widget/row.rs @@ -5,11 +5,12 @@ use crate::{ Style, Widget, }; -/// A container that places its contents horizontally. +/// A container that distributes its contents horizontally. /// /// A [`Row`] will try to fill the horizontal space of its container. /// /// [`Row`]: struct.Row.html +#[derive(Default)] pub struct Row<'a, Message, Renderer> { style: Style, spacing: u16, @@ -40,7 +41,7 @@ impl<'a, Message, Renderer> Row<'a, Message, Renderer> { /// Sets the horizontal spacing _between_ elements in pixels. /// - /// Custom margins per element do not exist in Coffee. You should use this + /// Custom margins per element do not exist in Iced. You should use this /// method instead! While less flexible, it helps you keep spacing between /// elements consistent. pub fn spacing(mut self, px: u16) -> Self { @@ -118,7 +119,7 @@ impl<'a, Message, Renderer> Row<'a, Message, Renderer> { /// Adds an [`Element`] to the [`Row`]. /// - /// [`Element`]: ../core/struct.Element.html + /// [`Element`]: ../struct.Element.html /// [`Row`]: struct.Row.html pub fn push<E>(mut self, child: E) -> Row<'a, Message, Renderer> where @@ -141,7 +142,7 @@ impl<'a, Message, Renderer> Widget<Message, Renderer> let mut style = node.0.style(); style.margin.end = - stretch::style::Dimension::Points(self.spacing as f32); + stretch::style::Dimension::Points(f32::from(self.spacing)); node.0.set_style(style); node @@ -196,12 +197,12 @@ impl<'a, Message, Renderer> Widget<Message, Renderer> cursor } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.style.hash(state); self.spacing.hash(state); for child in &self.children { - child.widget.hash(state); + child.widget.hash_layout(state); } } } diff --git a/src/widget/slider.rs b/src/widget/slider.rs index 75a0fdaf..c7adbb51 100644 --- a/src/widget/slider.rs +++ b/src/widget/slider.rs @@ -18,12 +18,11 @@ use crate::{ /// /// A [`Slider`] will try to fill the horizontal space of its container. /// -/// It implements [`Widget`] when the associated [`core::Renderer`] implements -/// the [`slider::Renderer`] trait. +/// It implements [`Widget`] when the associated `Renderer` implements the +/// [`slider::Renderer`] trait. /// /// [`Slider`]: struct.Slider.html -/// [`Widget`]: ../../core/trait.Widget.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [`Widget`]: ../trait.Widget.html /// [`slider::Renderer`]: trait.Renderer.html /// /// # Example @@ -39,6 +38,8 @@ use crate::{ /// /// Slider::new(state, 0.0..=100.0, value, Message::SliderChanged); /// ``` +/// +///  pub struct Slider<'a, Message> { state: &'a mut State, range: RangeInclusive<f32>, @@ -168,7 +169,7 @@ where ) } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.style.hash(state); } } @@ -200,11 +201,11 @@ impl State { /// The renderer of a [`Slider`]. /// -/// Your [`core::Renderer`] will need to implement this trait before being +/// Your [renderer] will need to implement this trait before being /// able to use a [`Slider`] in your user interface. /// /// [`Slider`]: struct.Slider.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [renderer]: ../../renderer/index.html pub trait Renderer { /// Draws a [`Slider`]. /// @@ -221,7 +222,7 @@ pub trait Renderer { fn draw( &mut self, cursor_position: Point, - bounds: Rectangle<f32>, + bounds: Rectangle, state: &State, range: RangeInclusive<f32>, value: f32, diff --git a/src/widget/text.rs b/src/widget/text.rs index e1ce8b16..7b62e5cb 100644 --- a/src/widget/text.rs +++ b/src/widget/text.rs @@ -5,13 +5,12 @@ use crate::{ use std::hash::Hash; -/// A fragment of text. +/// A fragment of text with a generic `Color`. /// -/// It implements [`Widget`] when the associated [`core::Renderer`] implements -/// the [`text::Renderer`] trait. +/// It implements [`Widget`] when the associated `Renderer` implements the +/// [`text::Renderer`] trait. /// -/// [`Widget`]: ../../core/trait.Widget.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [`Widget`]: ../trait.Widget.html /// [`text::Renderer`]: trait.Renderer.html /// /// # Example @@ -19,24 +18,26 @@ use std::hash::Hash; /// ``` /// use iced::Text; /// -/// Text::<(f32, f32, f32)>::new("I <3 iced!") +/// #[derive(Debug, Clone, Copy)] +/// pub enum Color { +/// Black, +/// } +/// +/// Text::new("I <3 iced!") /// .size(40) -/// .color((0.0, 0.0, 1.0)); +/// .color(Color::Black); /// ``` #[derive(Debug, Clone)] pub struct Text<Color> { content: String, size: u16, - color: Color, + color: Option<Color>, style: Style, horizontal_alignment: HorizontalAlignment, vertical_alignment: VerticalAlignment, } -impl<Color> Text<Color> -where - Color: Default, -{ +impl<Color> Text<Color> { /// Create a new fragment of [`Text`] with the given contents. /// /// [`Text`]: struct.Text.html @@ -44,7 +45,7 @@ where Text { content: String::from(label), size: 20, - color: Color::default(), + color: None, style: Style::default().fill_width(), horizontal_alignment: HorizontalAlignment::Left, vertical_alignment: VerticalAlignment::Top, @@ -59,12 +60,11 @@ where self } - /// Sets the [`Color`] of the [`Text`]. + /// Sets the `Color` of the [`Text`]. /// /// [`Text`]: struct.Text.html - /// [`Color`]: ../../../graphics/struct.Color.html pub fn color(mut self, color: Color) -> Self { - self.color = color; + self.color = Some(color); self } @@ -87,7 +87,7 @@ where /// Sets the [`HorizontalAlignment`] of the [`Text`]. /// /// [`Text`]: struct.Text.html - /// [`HorizontalAlignment`]: ../../../graphics/enum.HorizontalAlignment.html + /// [`HorizontalAlignment`]: enum.HorizontalAlignment.html pub fn horizontal_alignment( mut self, alignment: HorizontalAlignment, @@ -99,7 +99,7 @@ where /// Sets the [`VerticalAlignment`] of the [`Text`]. /// /// [`Text`]: struct.Text.html - /// [`VerticalAlignment`]: ../../../graphics/enum.VerticalAlignment.html + /// [`VerticalAlignment`]: enum.VerticalAlignment.html pub fn vertical_alignment(mut self, alignment: VerticalAlignment) -> Self { self.vertical_alignment = alignment; self @@ -112,7 +112,7 @@ where Renderer: self::Renderer<Color>, { fn node(&self, renderer: &Renderer) -> Node { - renderer.node(self.style, &self.content, self.size as f32) + renderer.node(self.style, &self.content, f32::from(self.size)) } fn draw( @@ -124,7 +124,7 @@ where renderer.draw( layout.bounds(), &self.content, - self.size as f32, + f32::from(self.size), self.color, self.horizontal_alignment, self.vertical_alignment, @@ -133,7 +133,7 @@ where MouseCursor::OutOfBounds } - fn hash(&self, state: &mut Hasher) { + fn hash_layout(&self, state: &mut Hasher) { self.style.hash(state); self.content.hash(state); @@ -141,13 +141,14 @@ where } } -/// The renderer of a [`Text`] fragment. +/// The renderer of a [`Text`] fragment with a generic `Color`. /// -/// Your [`core::Renderer`] will need to implement this trait before being -/// able to use a [`Text`] in your user interface. +/// Your [renderer] will need to implement this trait before being +/// able to use [`Text`] in your [`UserInterface`]. /// /// [`Text`]: struct.Text.html -/// [`core::Renderer`]: ../../core/trait.Renderer.html +/// [renderer]: ../../renderer/index.html +/// [`UserInterface`]: ../../struct.UserInterface.html pub trait Renderer<Color> { /// Creates a [`Node`] with the given [`Style`] for the provided [`Text`] /// contents and size. @@ -155,10 +156,10 @@ pub trait Renderer<Color> { /// You should probably use [`Node::with_measure`] to allow [`Text`] to /// adapt to the dimensions of its container. /// - /// [`Node`]: ../../core/struct.Node.html - /// [`Style`]: ../../core/struct.Style.html + /// [`Node`]: ../../struct.Node.html + /// [`Style`]: ../../struct.Style.html /// [`Text`]: struct.Text.html - /// [`Node::with_measure`]: ../../core/struct.Node.html#method.with_measure + /// [`Node::with_measure`]: ../../struct.Node.html#method.with_measure fn node(&self, style: Style, content: &str, size: f32) -> Node; /// Draws a [`Text`] fragment. @@ -172,14 +173,14 @@ pub trait Renderer<Color> { /// * the [`VerticalAlignment`] of the [`Text`] /// /// [`Text`]: struct.Text.html - /// [`HorizontalAlignment`]: ../../../graphics/enum.HorizontalAlignment.html - /// [`VerticalAlignment`]: ../../../graphics/enum.VerticalAlignment.html + /// [`HorizontalAlignment`]: enum.HorizontalAlignment.html + /// [`VerticalAlignment`]: enum.VerticalAlignment.html fn draw( &mut self, - bounds: Rectangle<f32>, + bounds: Rectangle, content: &str, size: f32, - color: Color, + color: Option<Color>, horizontal_alignment: HorizontalAlignment, vertical_alignment: VerticalAlignment, ); |