diff options
39 files changed, 794 insertions, 474 deletions
@@ -19,6 +19,7 @@ Once a step is completed, it is collapsed and added to this list: * [x] Custom styling ([#146]) * [x] Canvas for 2D graphics ([#193]) * [x] Basic overlay support ([#444]) + * [x] Animations [#31] [#24]: https://github.com/iced-rs/iced/issues/24 [#25]: https://github.com/iced-rs/iced/issues/25 @@ -29,6 +30,7 @@ Once a step is completed, it is collapsed and added to this list: [#146]: https://github.com/iced-rs/iced/pull/146 [#193]: https://github.com/iced-rs/iced/pull/193 [#444]: https://github.com/iced-rs/iced/pull/444 +[#31]: https://github.com/iced-rs/iced/issues/31 ### Multi-window support ([#27]) Open and control multiple windows at runtime. @@ -39,16 +41,7 @@ This approach should also allow us to perform custom optimizations for this part [#27]: https://github.com/iced-rs/iced/issues/27 -### Animations ([#31]) -Allow widgets to request a redraw at a specific time. - -This is a necessary feature to render loading spinners, a blinking text cursor, GIF images, etc. - -[`winit`] allows flexible control of its event loop. We may be able to use [`ControlFlow::WaitUntil`](https://docs.rs/winit/0.20.0-alpha3/winit/event_loop/enum.ControlFlow.html#variant.WaitUntil) for this purpose. - -[#31]: https://github.com/iced-rs/iced/issues/31 - -### Canvas widget for 3D graphics ([#32]) +### Canvas widget for 3D graphics (~~[#32]~~ [#343]) A widget to draw freely in 3D. It could be used to draw charts, implement a Paint clone, a CAD application, etc. As a first approach, we could expose the underlying renderer directly here, and couple this widget with it ([`wgpu`] for now). Once [`wgpu`] gets WebGL or WebGPU support, this widget will be able to run on the web too. The renderer primitive could be a simple texture that the widget draws to. @@ -56,6 +49,7 @@ As a first approach, we could expose the underlying renderer directly here, and In the long run, we could expose a renderer-agnostic abstraction to perform the drawing. [#32]: https://github.com/iced-rs/iced/issues/32 +[#343] https://github.com/iced-rs/iced/issues/343 ### Text shaping and font fallback ([#33]) [`wgpu_glyph`] uses [`glyph_brush`], which in turn uses [`rusttype`]. While the current implementation is able to layout text quite nicely, it does not perform any [text shaping]. diff --git a/core/src/mouse/interaction.rs b/core/src/mouse/interaction.rs index 57da93fe..072033fd 100644 --- a/core/src/mouse/interaction.rs +++ b/core/src/mouse/interaction.rs @@ -12,4 +12,5 @@ pub enum Interaction { Grabbing, ResizingHorizontally, ResizingVertically, + NotAllowed, } diff --git a/examples/component/src/main.rs b/examples/component/src/main.rs index bbf549e7..21c2747c 100644 --- a/examples/component/src/main.rs +++ b/examples/component/src/main.rs @@ -141,8 +141,8 @@ mod numeric_input { .map(u32::to_string) .as_deref() .unwrap_or(""), - Event::InputChanged, ) + .on_input(Event::InputChanged) .padding(10), button("+", Event::IncrementPressed), ] diff --git a/examples/download_progress/src/download.rs b/examples/download_progress/src/download.rs index 39dd843f..cd7647e8 100644 --- a/examples/download_progress/src/download.rs +++ b/examples/download_progress/src/download.rs @@ -18,10 +18,7 @@ pub struct Download<I> { url: String, } -async fn download<I: Copy>( - id: I, - state: State, -) -> (Option<(I, Progress)>, State) { +async fn download<I: Copy>(id: I, state: State) -> ((I, Progress), State) { match state { State::Ready(url) => { let response = reqwest::get(&url).await; @@ -30,7 +27,7 @@ async fn download<I: Copy>( Ok(response) => { if let Some(total) = response.content_length() { ( - Some((id, Progress::Started)), + (id, Progress::Started), State::Downloading { response, total, @@ -38,10 +35,10 @@ async fn download<I: Copy>( }, ) } else { - (Some((id, Progress::Errored)), State::Finished) + ((id, Progress::Errored), State::Finished) } } - Err(_) => (Some((id, Progress::Errored)), State::Finished), + Err(_) => ((id, Progress::Errored), State::Finished), } } State::Downloading { @@ -55,7 +52,7 @@ async fn download<I: Copy>( let percentage = (downloaded as f32 / total as f32) * 100.0; ( - Some((id, Progress::Advanced(percentage))), + (id, Progress::Advanced(percentage)), State::Downloading { response, total, @@ -63,8 +60,8 @@ async fn download<I: Copy>( }, ) } - Ok(None) => (Some((id, Progress::Finished)), State::Finished), - Err(_) => (Some((id, Progress::Errored)), State::Finished), + Ok(None) => ((id, Progress::Finished), State::Finished), + Err(_) => ((id, Progress::Errored), State::Finished), }, State::Finished => { // We do not let the stream die, as it would start a diff --git a/examples/integration_wgpu/src/controls.rs b/examples/integration_wgpu/src/controls.rs index 533cb6e2..42623b15 100644 --- a/examples/integration_wgpu/src/controls.rs +++ b/examples/integration_wgpu/src/controls.rs @@ -100,11 +100,10 @@ impl Program for Controls { .size(14) .style(Color::WHITE), ) - .push(text_input( - "Placeholder", - text, - Message::TextChanged, - )), + .push( + text_input("Placeholder", text) + .on_input(Message::TextChanged), + ), ), ) .into() diff --git a/examples/lazy/src/main.rs b/examples/lazy/src/main.rs index 6512106f..55b13bcf 100644 --- a/examples/lazy/src/main.rs +++ b/examples/lazy/src/main.rs @@ -214,12 +214,9 @@ impl Sandbox for App { column![ scrollable(options).height(Length::Fill), row![ - text_input( - "Add a new option", - &self.input, - Message::InputChanged, - ) - .on_submit(Message::AddItem(self.input.clone())), + text_input("Add a new option", &self.input) + .on_input(Message::InputChanged) + .on_submit(Message::AddItem(self.input.clone())), button(text(format!("Toggle Order ({})", self.order))) .on_press(Message::ToggleOrder) ] diff --git a/examples/modal/src/main.rs b/examples/modal/src/main.rs index 54555684..49038475 100644 --- a/examples/modal/src/main.rs +++ b/examples/modal/src/main.rs @@ -133,18 +133,16 @@ impl Application for App { column![ column![ text("Email").size(12), - text_input( - "abc@123.com", - &self.email, - Message::Email - ) - .on_submit(Message::Submit) - .padding(5), + text_input("abc@123.com", &self.email,) + .on_input(Message::Email) + .on_submit(Message::Submit) + .padding(5), ] .spacing(5), column![ text("Password").size(12), - text_input("", &self.password, Message::Password) + text_input("", &self.password) + .on_input(Message::Password) .on_submit(Message::Submit) .password() .padding(5), diff --git a/examples/qr_code/src/main.rs b/examples/qr_code/src/main.rs index d8041745..867ebfa4 100644 --- a/examples/qr_code/src/main.rs +++ b/examples/qr_code/src/main.rs @@ -49,13 +49,11 @@ impl Sandbox for QRGenerator { .size(70) .style(Color::from([0.5, 0.5, 0.5])); - let input = text_input( - "Type the data of your QR code here...", - &self.data, - Message::DataChanged, - ) - .size(30) - .padding(15); + let input = + text_input("Type the data of your QR code here...", &self.data) + .on_input(Message::DataChanged) + .size(30) + .padding(15); let mut content = column![title, input] .width(700) diff --git a/examples/styling/src/main.rs b/examples/styling/src/main.rs index 448c9792..e2015bac 100644 --- a/examples/styling/src/main.rs +++ b/examples/styling/src/main.rs @@ -90,13 +90,10 @@ impl Sandbox for Styling { }, ); - let text_input = text_input( - "Type something...", - &self.input_value, - Message::InputChanged, - ) - .padding(10) - .size(20); + let text_input = text_input("Type something...", &self.input_value) + .on_input(Message::InputChanged) + .padding(10) + .size(20); let button = button("Submit") .padding(10) diff --git a/examples/toast/src/main.rs b/examples/toast/src/main.rs index e74b3ee6..b4b4e007 100644 --- a/examples/toast/src/main.rs +++ b/examples/toast/src/main.rs @@ -119,13 +119,15 @@ impl Application for App { column![ subtitle( "Title", - text_input("", &self.editing.title, Message::Title) + text_input("", &self.editing.title) + .on_input(Message::Title) .on_submit(Message::Add) .into() ), subtitle( "Message", - text_input("", &self.editing.body, Message::Body) + text_input("", &self.editing.body) + .on_input(Message::Body) .on_submit(Message::Add) .into() ), diff --git a/examples/todos/src/main.rs b/examples/todos/src/main.rs index 6361667e..99cdb8f9 100644 --- a/examples/todos/src/main.rs +++ b/examples/todos/src/main.rs @@ -204,15 +204,12 @@ impl Application for Todos { .style(Color::from([0.5, 0.5, 0.5])) .horizontal_alignment(alignment::Horizontal::Center); - let input = text_input( - "What needs to be done?", - input_value, - Message::InputChanged, - ) - .id(INPUT_ID.clone()) - .padding(15) - .size(30) - .on_submit(Message::CreateTask); + let input = text_input("What needs to be done?", input_value) + .id(INPUT_ID.clone()) + .on_input(Message::InputChanged) + .on_submit(Message::CreateTask) + .padding(15) + .size(30); let controls = view_controls(tasks, *filter); let filtered_tasks = @@ -375,14 +372,12 @@ impl Task { .into() } TaskState::Editing => { - let text_input = text_input( - "Describe your task...", - &self.description, - TaskMessage::DescriptionEdited, - ) - .id(Self::text_input_id(i)) - .on_submit(TaskMessage::FinishEdition) - .padding(10); + let text_input = + text_input("Describe your task...", &self.description) + .id(Self::text_input_id(i)) + .on_input(TaskMessage::DescriptionEdited) + .on_submit(TaskMessage::FinishEdition) + .padding(10); row![ text_input, diff --git a/examples/tour/fonts/icons.ttf b/examples/tour/fonts/icons.ttf Binary files differnew file mode 100644 index 00000000..bfe8a24b --- /dev/null +++ b/examples/tour/fonts/icons.ttf diff --git a/examples/tour/src/main.rs b/examples/tour/src/main.rs index de063d00..16ee19c0 100644 --- a/examples/tour/src/main.rs +++ b/examples/tour/src/main.rs @@ -5,7 +5,7 @@ use iced::widget::{ scrollable, slider, text, text_input, toggler, vertical_space, }; use iced::widget::{Button, Column, Container, Slider}; -use iced::{Color, Element, Length, Renderer, Sandbox, Settings}; +use iced::{Color, Element, Font, Length, Renderer, Sandbox, Settings}; pub fn main() -> iced::Result { env_logger::init(); @@ -127,6 +127,7 @@ impl Steps { Step::TextInput { value: String::new(), is_secure: false, + is_showing_icon: false, }, Step::Debugger, Step::End, @@ -171,14 +172,32 @@ impl Steps { enum Step { Welcome, - Slider { value: u8 }, - RowsAndColumns { layout: Layout, spacing: u16 }, - Text { size: u16, color: Color }, - Radio { selection: Option<Language> }, - Toggler { can_continue: bool }, - Image { width: u16 }, + Slider { + value: u8, + }, + RowsAndColumns { + layout: Layout, + spacing: u16, + }, + Text { + size: u16, + color: Color, + }, + Radio { + selection: Option<Language>, + }, + Toggler { + can_continue: bool, + }, + Image { + width: u16, + }, Scrollable, - TextInput { value: String, is_secure: bool }, + TextInput { + value: String, + is_secure: bool, + is_showing_icon: bool, + }, Debugger, End, } @@ -194,6 +213,7 @@ pub enum StepMessage { ImageWidthChanged(u16), InputChanged(String), ToggleSecureInput(bool), + ToggleTextInputIcon(bool), DebugToggled(bool), TogglerChanged(bool), } @@ -256,6 +276,14 @@ impl<'a> Step { *can_continue = value; } } + StepMessage::ToggleTextInputIcon(toggle) => { + if let Step::TextInput { + is_showing_icon, .. + } = self + { + *is_showing_icon = toggle + } + } }; } @@ -303,9 +331,11 @@ impl<'a> Step { Self::rows_and_columns(*layout, *spacing) } Step::Scrollable => Self::scrollable(), - Step::TextInput { value, is_secure } => { - Self::text_input(value, *is_secure) - } + Step::TextInput { + value, + is_secure, + is_showing_icon, + } => Self::text_input(value, *is_secure, *is_showing_icon), Step::Debugger => Self::debugger(debug), Step::End => Self::end(), } @@ -530,14 +560,30 @@ impl<'a> Step { ) } - fn text_input(value: &str, is_secure: bool) -> Column<'a, StepMessage> { - let text_input = text_input( - "Type something to continue...", - value, - StepMessage::InputChanged, - ) - .padding(10) - .size(30); + fn text_input( + value: &str, + is_secure: bool, + is_showing_icon: bool, + ) -> Column<'a, StepMessage> { + const ICON_FONT: Font = Font::External { + name: "Icons", + bytes: include_bytes!("../fonts/icons.ttf"), + }; + + let mut text_input = text_input("Type something to continue...", value) + .on_input(StepMessage::InputChanged) + .padding(10) + .size(30); + + if is_showing_icon { + text_input = text_input.icon(text_input::Icon { + font: ICON_FONT, + code_point: '\u{E900}', + size: Some(35.0), + spacing: 10.0, + side: text_input::Side::Right, + }); + } Self::container("Text input") .push("Use a text input to ask for different kinds of information.") @@ -551,6 +597,11 @@ impl<'a> Step { is_secure, StepMessage::ToggleSecureInput, )) + .push(checkbox( + "Show icon", + is_showing_icon, + StepMessage::ToggleTextInputIcon, + )) .push( "A text input produces a message every time it changes. It is \ very easy to keep track of its contents:", diff --git a/examples/websocket/src/echo.rs b/examples/websocket/src/echo.rs index e74768a6..f9807172 100644 --- a/examples/websocket/src/echo.rs +++ b/examples/websocket/src/echo.rs @@ -13,63 +13,67 @@ use std::fmt; pub fn connect() -> Subscription<Event> { struct Connect; - subscription::unfold( + subscription::channel( std::any::TypeId::of::<Connect>(), - State::Disconnected, - |state| async move { - match state { - State::Disconnected => { - const ECHO_SERVER: &str = "ws://localhost:3030"; - - match async_tungstenite::tokio::connect_async(ECHO_SERVER) + 100, + |mut output| async move { + let mut state = State::Disconnected; + + loop { + match &mut state { + State::Disconnected => { + const ECHO_SERVER: &str = "ws://127.0.0.1:3030"; + + match async_tungstenite::tokio::connect_async( + ECHO_SERVER, + ) .await - { - Ok((websocket, _)) => { - let (sender, receiver) = mpsc::channel(100); - - ( - Some(Event::Connected(Connection(sender))), - State::Connected(websocket, receiver), - ) - } - Err(_) => { - tokio::time::sleep( - tokio::time::Duration::from_secs(1), - ) - .await; + { + Ok((websocket, _)) => { + let (sender, receiver) = mpsc::channel(100); + + let _ = output + .send(Event::Connected(Connection(sender))) + .await; - (Some(Event::Disconnected), State::Disconnected) + state = State::Connected(websocket, receiver); + } + Err(_) => { + tokio::time::sleep( + tokio::time::Duration::from_secs(1), + ) + .await; + + let _ = output.send(Event::Disconnected).await; + } } } - } - State::Connected(mut websocket, mut input) => { - let mut fused_websocket = websocket.by_ref().fuse(); - - futures::select! { - received = fused_websocket.select_next_some() => { - match received { - Ok(tungstenite::Message::Text(message)) => { - ( - Some(Event::MessageReceived(Message::User(message))), - State::Connected(websocket, input) - ) - } - Ok(_) => { - (None, State::Connected(websocket, input)) - } - Err(_) => { - (Some(Event::Disconnected), State::Disconnected) + State::Connected(websocket, input) => { + let mut fused_websocket = websocket.by_ref().fuse(); + + futures::select! { + received = fused_websocket.select_next_some() => { + match received { + Ok(tungstenite::Message::Text(message)) => { + let _ = output.send(Event::MessageReceived(Message::User(message))).await; + } + Err(_) => { + let _ = output.send(Event::Disconnected).await; + + state = State::Disconnected; + } + Ok(_) => continue, } } - } - message = input.select_next_some() => { - let result = websocket.send(tungstenite::Message::Text(message.to_string())).await; + message = input.select_next_some() => { + let result = websocket.send(tungstenite::Message::Text(message.to_string())).await; + + if result.is_err() { + let _ = output.send(Event::Disconnected).await; - if result.is_ok() { - (None, State::Connected(websocket, input)) - } else { - (Some(Event::Disconnected), State::Disconnected) + state = State::Disconnected; + } } } } diff --git a/examples/websocket/src/main.rs b/examples/websocket/src/main.rs index e617b8ce..920189f5 100644 --- a/examples/websocket/src/main.rs +++ b/examples/websocket/src/main.rs @@ -125,12 +125,9 @@ impl Application for WebSocket { }; let new_message_input = { - let mut input = text_input( - "Type a message...", - &self.new_message, - Message::NewMessageChanged, - ) - .padding(10); + let mut input = text_input("Type a message...", &self.new_message) + .on_input(Message::NewMessageChanged) + .padding(10); let mut button = button( text("Send") diff --git a/native/Cargo.toml b/native/Cargo.toml index 3f92783e..1eedf0da 100644 --- a/native/Cargo.toml +++ b/native/Cargo.toml @@ -14,6 +14,7 @@ debug = [] twox-hash = { version = "1.5", default-features = false } unicode-segmentation = "1.6" num-traits = "0.2" +thiserror = "1" [dependencies.iced_core] version = "0.8" diff --git a/native/src/subscription.rs b/native/src/subscription.rs index 16e78e82..0ff5e320 100644 --- a/native/src/subscription.rs +++ b/native/src/subscription.rs @@ -3,6 +3,8 @@ use crate::event::{self, Event}; use crate::window; use crate::Hasher; +use iced_futures::futures::channel::mpsc; +use iced_futures::futures::never::Never; use iced_futures::futures::{self, Future, Stream}; use iced_futures::{BoxStream, MaybeSend}; @@ -133,6 +135,27 @@ where /// [`Stream`] that will call the provided closure to produce every `Message`. /// /// The `id` will be used to uniquely identify the [`Subscription`]. +pub fn unfold<I, T, Fut, Message>( + id: I, + initial: T, + mut f: impl FnMut(T) -> Fut + MaybeSend + Sync + 'static, +) -> Subscription<Message> +where + I: Hash + 'static, + T: MaybeSend + 'static, + Fut: Future<Output = (Message, T)> + MaybeSend + 'static, + Message: 'static + MaybeSend, +{ + use futures::future::FutureExt; + + run_with_id( + id, + futures::stream::unfold(initial, move |state| f(state).map(Some)), + ) +} + +/// Creates a [`Subscription`] that publishes the events sent from a [`Future`] +/// to an [`mpsc::Sender`] with the given bounds. /// /// # Creating an asynchronous worker with bidirectional communication /// You can leverage this helper to create a [`Subscription`] that spawns @@ -145,6 +168,7 @@ where /// ``` /// use iced_native::subscription::{self, Subscription}; /// use iced_native::futures::channel::mpsc; +/// use iced_native::futures::sink::SinkExt; /// /// pub enum Event { /// Ready(mpsc::Sender<Input>), @@ -165,27 +189,35 @@ where /// fn some_worker() -> Subscription<Event> { /// struct SomeWorker; /// -/// subscription::unfold(std::any::TypeId::of::<SomeWorker>(), State::Starting, |state| async move { -/// match state { -/// State::Starting => { -/// // Create channel -/// let (sender, receiver) = mpsc::channel(100); +/// subscription::channel(std::any::TypeId::of::<SomeWorker>(), 100, |mut output| async move { +/// let mut state = State::Starting; /// -/// (Some(Event::Ready(sender)), State::Ready(receiver)) -/// } -/// State::Ready(mut receiver) => { -/// use iced_native::futures::StreamExt; +/// loop { +/// match &mut state { +/// State::Starting => { +/// // Create channel +/// let (sender, receiver) = mpsc::channel(100); +/// +/// // Send the sender back to the application +/// output.send(Event::Ready(sender)).await; +/// +/// // We are ready to receive messages +/// state = State::Ready(receiver); +/// } +/// State::Ready(receiver) => { +/// use iced_native::futures::StreamExt; /// -/// // Read next input sent from `Application` -/// let input = receiver.select_next_some().await; +/// // Read next input sent from `Application` +/// let input = receiver.select_next_some().await; /// -/// match input { -/// Input::DoSomeWork => { -/// // Do some async work... +/// match input { +/// Input::DoSomeWork => { +/// // Do some async work... /// -/// // Finally, we can optionally return a message to tell the -/// // `Application` the work is done -/// (Some(Event::WorkFinished), State::Ready(receiver)) +/// // Finally, we can optionally produce a message to tell the +/// // `Application` the work is done +/// output.send(Event::WorkFinished).await; +/// } /// } /// } /// } @@ -198,25 +230,28 @@ where /// connection open. /// /// [`websocket`]: https://github.com/iced-rs/iced/tree/0.8/examples/websocket -pub fn unfold<I, T, Fut, Message>( +pub fn channel<I, Fut, Message>( id: I, - initial: T, - mut f: impl FnMut(T) -> Fut + MaybeSend + Sync + 'static, + size: usize, + f: impl Fn(mpsc::Sender<Message>) -> Fut + MaybeSend + Sync + 'static, ) -> Subscription<Message> where I: Hash + 'static, - T: MaybeSend + 'static, - Fut: Future<Output = (Option<Message>, T)> + MaybeSend + 'static, + Fut: Future<Output = Never> + MaybeSend + 'static, Message: 'static + MaybeSend, { - use futures::future::{self, FutureExt}; - use futures::stream::StreamExt; + use futures::stream::{self, StreamExt}; - run_with_id( + Subscription::from_recipe(Runner { id, - futures::stream::unfold(initial, move |state| f(state).map(Some)) - .filter_map(future::ready), - ) + spawn: move |_| { + let (sender, receiver) = mpsc::channel(size); + + let runner = stream::once(f(sender)).map(|_| unreachable!()); + + stream::select(receiver, runner) + }, + }) } struct Runner<I, F, S, Message> diff --git a/native/src/widget/checkbox.rs b/native/src/widget/checkbox.rs index 9b69e574..ad05a8e7 100644 --- a/native/src/widget/checkbox.rs +++ b/native/src/widget/checkbox.rs @@ -14,17 +14,6 @@ use crate::{ pub use iced_style::checkbox::{Appearance, StyleSheet}; -/// The icon in a [`Checkbox`]. -#[derive(Debug, Clone, PartialEq)] -pub struct Icon<Font> { - /// Font that will be used to display the `code_point`, - pub font: Font, - /// The unicode code point that will be used as the icon. - pub code_point: char, - /// Font size of the content. - pub size: Option<f32>, -} - /// A box that can be checked. /// /// # Example @@ -319,3 +308,14 @@ where Element::new(checkbox) } } + +/// The icon in a [`Checkbox`]. +#[derive(Debug, Clone, PartialEq)] +pub struct Icon<Font> { + /// Font that will be used to display the `code_point`, + pub font: Font, + /// The unicode code point that will be used as the icon. + pub code_point: char, + /// Font size of the content. + pub size: Option<f32>, +} diff --git a/native/src/widget/helpers.rs b/native/src/widget/helpers.rs index d13eca75..b25e064d 100644 --- a/native/src/widget/helpers.rs +++ b/native/src/widget/helpers.rs @@ -147,7 +147,7 @@ where Renderer::Theme: widget::radio::StyleSheet, V: Copy + Eq, { - widget::Radio::new(value, label, selected, on_click) + widget::Radio::new(label, value, selected, on_click) } /// Creates a new [`Toggler`]. @@ -171,14 +171,13 @@ where pub fn text_input<'a, Message, Renderer>( placeholder: &str, value: &str, - on_change: impl Fn(String) -> Message + 'a, ) -> widget::TextInput<'a, Message, Renderer> where Message: Clone, Renderer: crate::text::Renderer, Renderer::Theme: widget::text_input::StyleSheet, { - widget::TextInput::new(placeholder, value, on_change) + widget::TextInput::new(placeholder, value) } /// Creates a new [`Slider`]. diff --git a/native/src/widget/radio.rs b/native/src/widget/radio.rs index 9daddfbc..3ca041bf 100644 --- a/native/src/widget/radio.rs +++ b/native/src/widget/radio.rs @@ -21,10 +21,13 @@ pub use iced_style::radio::{Appearance, StyleSheet}; /// # type Radio<Message> = /// # iced_native::widget::Radio<Message, iced_native::renderer::Null>; /// # +/// # use iced_native::column; /// #[derive(Debug, Clone, Copy, PartialEq, Eq)] /// pub enum Choice { /// A, /// B, +/// C, +/// All, /// } /// /// #[derive(Debug, Clone, Copy)] @@ -34,12 +37,36 @@ pub use iced_style::radio::{Appearance, StyleSheet}; /// /// let selected_choice = Some(Choice::A); /// -/// Radio::new(Choice::A, "This is A", selected_choice, Message::RadioSelected); +/// let a = Radio::new( +/// "A", +/// Choice::A, +/// selected_choice, +/// Message::RadioSelected, +/// ); /// -/// Radio::new(Choice::B, "This is B", selected_choice, Message::RadioSelected); -/// ``` +/// let b = Radio::new( +/// "B", +/// Choice::B, +/// selected_choice, +/// Message::RadioSelected, +/// ); +/// +/// let c = Radio::new( +/// "C", +/// Choice::C, +/// selected_choice, +/// Message::RadioSelected, +/// ); +/// +/// let all = Radio::new( +/// "All of the above", +/// Choice::All, +/// selected_choice, +/// Message::RadioSelected +/// ); /// -/// 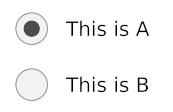 +/// let content = column![a, b, c, all]; +/// ``` #[allow(missing_debug_implementations)] pub struct Radio<Message, Renderer> where @@ -78,8 +105,8 @@ where /// * a function that will be called when the [`Radio`] is selected. It /// receives the value of the radio and must produce a `Message`. pub fn new<F, V>( - value: V, label: impl Into<String>, + value: V, selected: Option<V>, f: F, ) -> Self diff --git a/native/src/widget/slider.rs b/native/src/widget/slider.rs index d3715b1c..69c06140 100644 --- a/native/src/widget/slider.rs +++ b/native/src/widget/slider.rs @@ -8,13 +8,15 @@ use crate::renderer; use crate::touch; use crate::widget::tree::{self, Tree}; use crate::{ - Background, Clipboard, Color, Element, Layout, Length, Pixels, Point, - Rectangle, Shell, Size, Widget, + Clipboard, Color, Element, Layout, Length, Pixels, Point, Rectangle, Shell, + Size, Widget, }; use std::ops::RangeInclusive; -pub use iced_style::slider::{Appearance, Handle, HandleShape, StyleSheet}; +pub use iced_style::slider::{ + Appearance, Handle, HandleShape, Rail, StyleSheet, +}; /// An horizontal bar and a handle that selects a single value from a range of /// values. @@ -368,38 +370,6 @@ pub fn draw<T, R>( style_sheet.active(style) }; - let rail_y = bounds.y + (bounds.height / 2.0).round(); - - renderer.fill_quad( - renderer::Quad { - bounds: Rectangle { - x: bounds.x, - y: rail_y - 1.0, - width: bounds.width, - height: 2.0, - }, - border_radius: 0.0.into(), - border_width: 0.0, - border_color: Color::TRANSPARENT, - }, - style.rail_colors.0, - ); - - renderer.fill_quad( - renderer::Quad { - bounds: Rectangle { - x: bounds.x, - y: rail_y + 1.0, - width: bounds.width, - height: 2.0, - }, - border_radius: 0.0.into(), - border_width: 0.0, - border_color: Color::TRANSPARENT, - }, - Background::Color(style.rail_colors.1), - ); - let (handle_width, handle_height, handle_border_radius) = match style .handle .shape @@ -418,17 +388,61 @@ pub fn draw<T, R>( (start.into() as f32, end.into() as f32) }; - let handle_offset = if range_start >= range_end { + let offset = if range_start >= range_end { 0.0 } else { (bounds.width - handle_width) * (value - range_start) / (range_end - range_start) }; + let rail_y = bounds.y + bounds.height / 2.0; + + renderer.fill_quad( + renderer::Quad { + bounds: Rectangle { + x: bounds.x, + y: rail_y - style.rail.width / 2.0, + width: offset, + height: style.rail.width, + }, + border_radius: [ + style.rail.border_radius, + 0.0, + 0.0, + style.rail.border_radius, + ] + .into(), + border_width: 0.0, + border_color: Color::TRANSPARENT, + }, + style.rail.colors.0, + ); + + renderer.fill_quad( + renderer::Quad { + bounds: Rectangle { + x: bounds.x + offset, + y: rail_y - style.rail.width / 2.0, + width: bounds.width - offset, + height: style.rail.width, + }, + border_radius: [ + 0.0, + style.rail.border_radius, + style.rail.border_radius, + 0.0, + ] + .into(), + border_width: 0.0, + border_color: Color::TRANSPARENT, + }, + style.rail.colors.1, + ); + renderer.fill_quad( renderer::Quad { bounds: Rectangle { - x: bounds.x + handle_offset.round(), + x: bounds.x + offset.round(), y: rail_y - handle_height / 2.0, width: handle_width, height: handle_height, diff --git a/native/src/widget/text_input.rs b/native/src/widget/text_input.rs index ee0473ea..8627aa98 100644 --- a/native/src/widget/text_input.rs +++ b/native/src/widget/text_input.rs @@ -46,8 +46,8 @@ pub use iced_style::text_input::{Appearance, StyleSheet}; /// let input = TextInput::new( /// "This is the placeholder...", /// value, -/// Message::TextInputChanged, /// ) +/// .on_input(Message::TextInputChanged) /// .padding(10); /// ``` /// 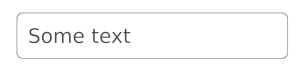 @@ -65,9 +65,10 @@ where width: Length, padding: Padding, size: Option<f32>, - on_change: Box<dyn Fn(String) -> Message + 'a>, + on_input: Option<Box<dyn Fn(String) -> Message + 'a>>, on_paste: Option<Box<dyn Fn(String) -> Message + 'a>>, on_submit: Option<Message>, + icon: Option<Icon<Renderer::Font>>, style: <Renderer::Theme as StyleSheet>::Style, } @@ -81,12 +82,8 @@ where /// /// It expects: /// - a placeholder, - /// - the current value, and - /// - a function that produces a message when the [`TextInput`] changes. - pub fn new<F>(placeholder: &str, value: &str, on_change: F) -> Self - where - F: 'a + Fn(String) -> Message, - { + /// - the current value + pub fn new(placeholder: &str, value: &str) -> Self { TextInput { id: None, placeholder: String::from(placeholder), @@ -96,9 +93,10 @@ where width: Length::Fill, padding: Padding::new(5.0), size: None, - on_change: Box::new(on_change), + on_input: None, on_paste: None, on_submit: None, + icon: None, style: Default::default(), } } @@ -115,6 +113,25 @@ where self } + /// Sets the message that should be produced when some text is typed into + /// the [`TextInput`]. + /// + /// If this method is not called, the [`TextInput`] will be disabled. + pub fn on_input<F>(mut self, callback: F) -> Self + where + F: 'a + Fn(String) -> Message, + { + self.on_input = Some(Box::new(callback)); + self + } + + /// Sets the message that should be produced when the [`TextInput`] is + /// focused and the enter key is pressed. + pub fn on_submit(mut self, message: Message) -> Self { + self.on_submit = Some(message); + self + } + /// Sets the message that should be produced when some text is pasted into /// the [`TextInput`]. pub fn on_paste( @@ -132,6 +149,13 @@ where self.font = font; self } + + /// Sets the [`Icon`] of the [`TextInput`]. + pub fn icon(mut self, icon: Icon<Renderer::Font>) -> Self { + self.icon = Some(icon); + self + } + /// Sets the width of the [`TextInput`]. pub fn width(mut self, width: impl Into<Length>) -> Self { self.width = width.into(); @@ -150,13 +174,6 @@ where self } - /// Sets the message that should be produced when the [`TextInput`] is - /// focused and the enter key is pressed. - pub fn on_submit(mut self, message: Message) -> Self { - self.on_submit = Some(message); - self - } - /// Sets the style of the [`TextInput`]. pub fn style( mut self, @@ -189,7 +206,9 @@ where &self.placeholder, self.size, &self.font, + self.on_input.is_none(), self.is_secure, + self.icon.as_ref(), &self.style, ) } @@ -210,6 +229,18 @@ where tree::State::new(State::new()) } + fn diff(&self, tree: &mut Tree) { + let state = tree.state.downcast_mut::<State>(); + + // Unfocus text input if it becomes disabled + if self.on_input.is_none() { + state.last_click = None; + state.is_focused = None; + state.is_pasting = None; + state.is_dragging = false; + } + } + fn width(&self) -> Length { self.width } @@ -223,7 +254,14 @@ where renderer: &Renderer, limits: &layout::Limits, ) -> layout::Node { - layout(renderer, limits, self.width, self.padding, self.size) + layout( + renderer, + limits, + self.width, + self.padding, + self.size, + self.icon.as_ref(), + ) } fn operate( @@ -260,7 +298,7 @@ where self.size, &self.font, self.is_secure, - self.on_change.as_ref(), + self.on_input.as_deref(), self.on_paste.as_deref(), &self.on_submit, || tree.state.downcast_mut::<State>(), @@ -287,7 +325,9 @@ where &self.placeholder, self.size, &self.font, + self.on_input.is_none(), self.is_secure, + self.icon.as_ref(), &self.style, ) } @@ -300,7 +340,7 @@ where _viewport: &Rectangle, _renderer: &Renderer, ) -> mouse::Interaction { - mouse_interaction(layout, cursor_position) + mouse_interaction(layout, cursor_position, self.on_input.is_none()) } } @@ -318,6 +358,30 @@ where } } +/// The content of the [`Icon`]. +#[derive(Debug, Clone)] +pub struct Icon<Font> { + /// The font that will be used to display the `code_point`. + pub font: Font, + /// The unicode code point that will be used as the icon. + pub code_point: char, + /// The font size of the content. + pub size: Option<f32>, + /// The spacing between the [`Icon`] and the text in a [`TextInput`]. + pub spacing: f32, + /// The side of a [`TextInput`] where to display the [`Icon`]. + pub side: Side, +} + +/// The side of a [`TextInput`]. +#[derive(Debug, Clone)] +pub enum Side { + /// The left side of a [`TextInput`]. + Left, + /// The right side of a [`TextInput`]. + Right, +} + /// The identifier of a [`TextInput`]. #[derive(Debug, Clone, PartialEq, Eq, Hash)] pub struct Id(widget::Id); @@ -380,6 +444,7 @@ pub fn layout<Renderer>( width: Length, padding: Padding, size: Option<f32>, + icon: Option<&Icon<Renderer::Font>>, ) -> layout::Node where Renderer: text::Renderer, @@ -389,10 +454,51 @@ where let padding = padding.fit(Size::ZERO, limits.max()); let limits = limits.width(width).pad(padding).height(text_size); - let mut text = layout::Node::new(limits.resolve(Size::ZERO)); - text.move_to(Point::new(padding.left, padding.top)); + let text_bounds = limits.resolve(Size::ZERO); + + if let Some(icon) = icon { + let icon_width = renderer.measure_width( + &icon.code_point.to_string(), + icon.size.unwrap_or_else(|| renderer.default_size()), + icon.font.clone(), + ); + + let mut text_node = layout::Node::new( + text_bounds - Size::new(icon_width + icon.spacing, 0.0), + ); + + let mut icon_node = + layout::Node::new(Size::new(icon_width, text_bounds.height)); + + match icon.side { + Side::Left => { + text_node.move_to(Point::new( + padding.left + icon_width + icon.spacing, + padding.top, + )); + + icon_node.move_to(Point::new(padding.left, padding.top)); + } + Side::Right => { + text_node.move_to(Point::new(padding.left, padding.top)); + + icon_node.move_to(Point::new( + padding.left + text_bounds.width - icon_width, + padding.top, + )); + } + }; + + layout::Node::with_children( + text_bounds.pad(padding), + vec![text_node, icon_node], + ) + } else { + let mut text = layout::Node::new(text_bounds); + text.move_to(Point::new(padding.left, padding.top)); - layout::Node::with_children(text.size().pad(padding), vec![text]) + layout::Node::with_children(text_bounds.pad(padding), vec![text]) + } } /// Processes an [`Event`] and updates the [`State`] of a [`TextInput`] @@ -408,7 +514,7 @@ pub fn update<'a, Message, Renderer>( size: Option<f32>, font: &Renderer::Font, is_secure: bool, - on_change: &dyn Fn(String) -> Message, + on_input: Option<&dyn Fn(String) -> Message>, on_paste: Option<&dyn Fn(String) -> Message>, on_submit: &Option<Message>, state: impl FnOnce() -> &'a mut State, @@ -421,7 +527,8 @@ where Event::Mouse(mouse::Event::ButtonPressed(mouse::Button::Left)) | Event::Touch(touch::Event::FingerPressed { .. }) => { let state = state(); - let is_clicked = layout.bounds().contains(cursor_position); + let is_clicked = + layout.bounds().contains(cursor_position) && on_input.is_some(); state.is_focused = if is_clicked { state.is_focused.or_else(|| { @@ -551,6 +658,8 @@ where let state = state(); if let Some(focus) = &mut state.is_focused { + let Some(on_input) = on_input else { return event::Status::Ignored }; + if state.is_pasting.is_none() && !state.keyboard_modifiers.command() && !c.is_control() @@ -559,7 +668,7 @@ where editor.insert(c); - let message = (on_change)(editor.contents()); + let message = (on_input)(editor.contents()); shell.publish(message); focus.updated_at = Instant::now(); @@ -572,6 +681,8 @@ where let state = state(); if let Some(focus) = &mut state.is_focused { + let Some(on_input) = on_input else { return event::Status::Ignored }; + let modifiers = state.keyboard_modifiers; focus.updated_at = Instant::now(); @@ -597,7 +708,7 @@ where let mut editor = Editor::new(value, &mut state.cursor); editor.backspace(); - let message = (on_change)(editor.contents()); + let message = (on_input)(editor.contents()); shell.publish(message); } keyboard::KeyCode::Delete => { @@ -617,7 +728,7 @@ where let mut editor = Editor::new(value, &mut state.cursor); editor.delete(); - let message = (on_change)(editor.contents()); + let message = (on_input)(editor.contents()); shell.publish(message); } keyboard::KeyCode::Left => { @@ -692,7 +803,7 @@ where let mut editor = Editor::new(value, &mut state.cursor); editor.delete(); - let message = (on_change)(editor.contents()); + let message = (on_input)(editor.contents()); shell.publish(message); } keyboard::KeyCode::V => { @@ -719,7 +830,7 @@ where let message = if let Some(paste) = &on_paste { (paste)(editor.contents()) } else { - (on_change)(editor.contents()) + (on_input)(editor.contents()) }; shell.publish(message); @@ -813,7 +924,9 @@ pub fn draw<Renderer>( placeholder: &str, size: Option<f32>, font: &Renderer::Font, + is_disabled: bool, is_secure: bool, + icon: Option<&Icon<Renderer::Font>>, style: &<Renderer::Theme as StyleSheet>::Style, ) where Renderer: text::Renderer, @@ -823,11 +936,15 @@ pub fn draw<Renderer>( let value = secure_value.as_ref().unwrap_or(value); let bounds = layout.bounds(); - let text_bounds = layout.children().next().unwrap().bounds(); + + let mut children_layout = layout.children(); + let text_bounds = children_layout.next().unwrap().bounds(); let is_mouse_over = bounds.contains(cursor_position); - let appearance = if state.is_focused() { + let appearance = if is_disabled { + theme.disabled(style) + } else if state.is_focused() { theme.focused(style) } else if is_mouse_over { theme.hovered(style) @@ -845,6 +962,20 @@ pub fn draw<Renderer>( appearance.background, ); + if let Some(icon) = icon { + let icon_layout = children_layout.next().unwrap(); + + renderer.fill_text(Text { + content: &icon.code_point.to_string(), + size: icon.size.unwrap_or_else(|| renderer.default_size()), + font: icon.font.clone(), + color: appearance.icon_color, + bounds: icon_layout.bounds(), + horizontal_alignment: alignment::Horizontal::Left, + vertical_alignment: alignment::Vertical::Top, + }); + } + let text = value.to_string(); let size = size.unwrap_or_else(|| renderer.default_size()); @@ -956,6 +1087,8 @@ pub fn draw<Renderer>( content: if text.is_empty() { placeholder } else { &text }, color: if text.is_empty() { theme.placeholder_color(style) + } else if is_disabled { + theme.disabled_color(style) } else { theme.value_color(style) }, @@ -984,9 +1117,14 @@ pub fn draw<Renderer>( pub fn mouse_interaction( layout: Layout<'_>, cursor_position: Point, + is_disabled: bool, ) -> mouse::Interaction { if layout.bounds().contains(cursor_position) { - mouse::Interaction::Text + if is_disabled { + mouse::Interaction::NotAllowed + } else { + mouse::Interaction::Text + } } else { mouse::Interaction::default() } diff --git a/native/src/widget/vertical_slider.rs b/native/src/widget/vertical_slider.rs index f1687e38..a06a200f 100644 --- a/native/src/widget/vertical_slider.rs +++ b/native/src/widget/vertical_slider.rs @@ -8,8 +8,8 @@ pub use iced_style::slider::{Appearance, Handle, HandleShape, StyleSheet}; use crate::event::{self, Event}; use crate::widget::tree::{self, Tree}; use crate::{ - layout, mouse, renderer, touch, Background, Clipboard, Color, Element, - Layout, Length, Pixels, Point, Rectangle, Shell, Size, Widget, + layout, mouse, renderer, touch, Clipboard, Color, Element, Layout, Length, + Pixels, Point, Rectangle, Shell, Size, Widget, }; /// An vertical bar and a handle that selects a single value from a range of @@ -363,38 +363,6 @@ pub fn draw<T, R>( style_sheet.active(style) }; - let rail_x = bounds.x + (bounds.width / 2.0).round(); - - renderer.fill_quad( - renderer::Quad { - bounds: Rectangle { - x: rail_x - 1.0, - y: bounds.y, - width: 2.0, - height: bounds.height, - }, - border_radius: 0.0.into(), - border_width: 0.0, - border_color: Color::TRANSPARENT, - }, - style.rail_colors.0, - ); - - renderer.fill_quad( - renderer::Quad { - bounds: Rectangle { - x: rail_x + 1.0, - y: bounds.y, - width: 2.0, - height: bounds.height, - }, - border_radius: 0.0.into(), - border_width: 0.0, - border_color: Color::TRANSPARENT, - }, - Background::Color(style.rail_colors.1), - ); - let (handle_width, handle_height, handle_border_radius) = match style .handle .shape @@ -413,18 +381,62 @@ pub fn draw<T, R>( (start.into() as f32, end.into() as f32) }; - let handle_offset = if range_start >= range_end { + let offset = if range_start >= range_end { 0.0 } else { (bounds.height - handle_width) * (value - range_end) / (range_start - range_end) }; + let rail_x = bounds.x + bounds.width / 2.0; + + renderer.fill_quad( + renderer::Quad { + bounds: Rectangle { + x: rail_x - style.rail.width / 2.0, + y: bounds.y, + width: style.rail.width, + height: offset, + }, + border_radius: [ + style.rail.border_radius, + style.rail.border_radius, + 0.0, + 0.0, + ] + .into(), + border_width: 0.0, + border_color: Color::TRANSPARENT, + }, + style.rail.colors.1, + ); + + renderer.fill_quad( + renderer::Quad { + bounds: Rectangle { + x: rail_x - style.rail.width / 2.0, + y: bounds.y + offset, + width: style.rail.width, + height: bounds.height - offset, + }, + border_radius: [ + 0.0, + 0.0, + style.rail.border_radius, + style.rail.border_radius, + ] + .into(), + border_width: 0.0, + border_color: Color::TRANSPARENT, + }, + style.rail.colors.0, + ); + renderer.fill_quad( renderer::Quad { bounds: Rectangle { - x: rail_x - (handle_height / 2.0), - y: bounds.y + handle_offset.round(), + x: rail_x - handle_height / 2.0, + y: bounds.y + offset.round(), width: handle_height, height: handle_width, }, diff --git a/native/src/window.rs b/native/src/window.rs index a5cdc8ce..1ae89dba 100644 --- a/native/src/window.rs +++ b/native/src/window.rs @@ -5,8 +5,11 @@ mod mode; mod redraw_request; mod user_attention; +pub mod icon; + pub use action::Action; pub use event::Event; +pub use icon::Icon; pub use mode::Mode; pub use redraw_request::RedrawRequest; pub use user_attention::UserAttention; diff --git a/native/src/window/action.rs b/native/src/window/action.rs index ce36d129..095a8eec 100644 --- a/native/src/window/action.rs +++ b/native/src/window/action.rs @@ -1,4 +1,4 @@ -use crate::window::{Mode, UserAttention}; +use crate::window::{Icon, Mode, UserAttention}; use iced_futures::MaybeSend; use std::fmt; @@ -78,6 +78,21 @@ pub enum Action<T> { ChangeAlwaysOnTop(bool), /// Fetch an identifier unique to the window. FetchId(Box<dyn FnOnce(u64) -> T + 'static>), + /// Changes the window [`Icon`]. + /// + /// On Windows and X11, this is typically the small icon in the top-left + /// corner of the titlebar. + /// + /// ## Platform-specific + /// + /// - **Web / Wayland / macOS:** Unsupported. + /// + /// - **Windows:** Sets `ICON_SMALL`. The base size for a window icon is 16x16, but it's + /// recommended to account for screen scaling and pick a multiple of that, i.e. 32x32. + /// + /// - **X11:** Has no universal guidelines for icon sizes, so you're at the whims of the WM. That + /// said, it's usually in the same ballpark as on Windows. + ChangeIcon(Icon), } impl<T> Action<T> { @@ -108,6 +123,7 @@ impl<T> Action<T> { Action::ChangeAlwaysOnTop(on_top) } Self::FetchId(o) => Action::FetchId(Box::new(move |s| f(o(s)))), + Self::ChangeIcon(icon) => Action::ChangeIcon(icon), } } } @@ -142,6 +158,9 @@ impl<T> fmt::Debug for Action<T> { write!(f, "Action::AlwaysOnTop({on_top})") } Self::FetchId(_) => write!(f, "Action::FetchId"), + Self::ChangeIcon(_icon) => { + write!(f, "Action::ChangeIcon(icon)") + } } } } diff --git a/native/src/window/icon.rs b/native/src/window/icon.rs new file mode 100644 index 00000000..31868ecf --- /dev/null +++ b/native/src/window/icon.rs @@ -0,0 +1,80 @@ +//! Change the icon of a window. +use crate::Size; + +use std::mem; + +/// Builds an [`Icon`] from its RGBA pixels in the sRGB color space. +pub fn from_rgba( + rgba: Vec<u8>, + width: u32, + height: u32, +) -> Result<Icon, Error> { + const PIXEL_SIZE: usize = mem::size_of::<u8>() * 4; + + if rgba.len() % PIXEL_SIZE != 0 { + return Err(Error::ByteCountNotDivisibleBy4 { + byte_count: rgba.len(), + }); + } + + let pixel_count = rgba.len() / PIXEL_SIZE; + + if pixel_count != (width * height) as usize { + return Err(Error::DimensionsVsPixelCount { + width, + height, + width_x_height: (width * height) as usize, + pixel_count, + }); + } + + Ok(Icon { + rgba, + size: Size::new(width, height), + }) +} + +/// An window icon normally used for the titlebar or taskbar. +#[derive(Debug, Clone)] +pub struct Icon { + rgba: Vec<u8>, + size: Size<u32>, +} + +impl Icon { + /// Returns the raw data of the [`Icon`]. + pub fn into_raw(self) -> (Vec<u8>, Size<u32>) { + (self.rgba, self.size) + } +} + +#[derive(Debug, thiserror::Error)] +/// An error produced when using [`Icon::from_rgba`] with invalid arguments. +pub enum Error { + /// Produced when the length of the `rgba` argument isn't divisible by 4, thus `rgba` can't be + /// safely interpreted as 32bpp RGBA pixels. + #[error( + "The provided RGBA data (with length {byte_count}) isn't divisible \ + by 4. Therefore, it cannot be safely interpreted as 32bpp RGBA pixels" + )] + ByteCountNotDivisibleBy4 { + /// The length of the provided RGBA data. + byte_count: usize, + }, + /// Produced when the number of pixels (`rgba.len() / 4`) isn't equal to `width * height`. + /// At least one of your arguments is incorrect. + #[error( + "The number of RGBA pixels ({pixel_count}) does not match the \ + provided dimensions ({width}x{height})." + )] + DimensionsVsPixelCount { + /// The provided width. + width: u32, + /// The provided height. + height: u32, + /// The product of `width` and `height`. + width_x_height: usize, + /// The amount of pixels of the provided RGBA data. + pixel_count: usize, + }, +} diff --git a/src/widget.rs b/src/widget.rs index e2b0537e..c0ac716f 100644 --- a/src/widget.rs +++ b/src/widget.rs @@ -124,7 +124,7 @@ pub mod text_input { //! Display fields that can be filled with text. pub use iced_native::widget::text_input::{ focus, move_cursor_to, move_cursor_to_end, move_cursor_to_front, - select_all, Appearance, Id, StyleSheet, + select_all, Appearance, Icon, Id, Side, StyleSheet, }; /// A field that can be filled with text. diff --git a/src/window/icon.rs b/src/window/icon.rs index 659d2b64..e09dd048 100644 --- a/src/window/icon.rs +++ b/src/window/icon.rs @@ -1,175 +1,66 @@ //! Attach an icon to the window of your application. -use std::fmt; +pub use crate::runtime::window::icon::*; + +use crate::runtime::window::icon; + use std::io; -#[cfg(feature = "image_rs")] +#[cfg(feature = "image")] use std::path::Path; -/// The icon of a window. -#[derive(Clone)] -pub struct Icon(iced_winit::winit::window::Icon); +/// Creates an icon from an image file. +/// +/// This will return an error in case the file is missing at run-time. You may prefer [`Self::from_file_data`] instead. +#[cfg(feature = "image")] +#[cfg_attr(docsrs, doc(cfg(feature = "image")))] +pub fn from_file<P: AsRef<Path>>(icon_path: P) -> Result<Icon, Error> { + let icon = image_rs::io::Reader::open(icon_path)?.decode()?.to_rgba8(); -impl fmt::Debug for Icon { - fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result { - f.debug_tuple("Icon").field(&format_args!("_")).finish() - } + Ok(icon::from_rgba(icon.to_vec(), icon.width(), icon.height())?) } -impl Icon { - /// Creates an icon from 32bpp RGBA data. - pub fn from_rgba( - rgba: Vec<u8>, - width: u32, - height: u32, - ) -> Result<Self, Error> { - let raw = - iced_winit::winit::window::Icon::from_rgba(rgba, width, height)?; - - Ok(Icon(raw)) - } - - /// Creates an icon from an image file. - /// - /// This will return an error in case the file is missing at run-time. You may prefer [`Self::from_file_data`] instead. - #[cfg(feature = "image_rs")] - pub fn from_file<P: AsRef<Path>>(icon_path: P) -> Result<Self, Error> { - let icon = image_rs::io::Reader::open(icon_path)?.decode()?.to_rgba8(); - - Self::from_rgba(icon.to_vec(), icon.width(), icon.height()) - } - - /// Creates an icon from the content of an image file. - /// - /// This content can be included in your application at compile-time, e.g. using the `include_bytes!` macro. \ - /// You can pass an explicit file format. Otherwise, the file format will be guessed at runtime. - #[cfg(feature = "image_rs")] - pub fn from_file_data( - data: &[u8], - explicit_format: Option<image_rs::ImageFormat>, - ) -> Result<Self, Error> { - let mut icon = image_rs::io::Reader::new(std::io::Cursor::new(data)); - let icon_with_format = match explicit_format { - Some(format) => { - icon.set_format(format); - icon - } - None => icon.with_guessed_format()?, - }; +/// Creates an icon from the content of an image file. +/// +/// This content can be included in your application at compile-time, e.g. using the `include_bytes!` macro. +/// You can pass an explicit file format. Otherwise, the file format will be guessed at runtime. +#[cfg(feature = "image")] +#[cfg_attr(docsrs, doc(cfg(feature = "image")))] +pub fn from_file_data( + data: &[u8], + explicit_format: Option<image_rs::ImageFormat>, +) -> Result<Icon, Error> { + let mut icon = image_rs::io::Reader::new(std::io::Cursor::new(data)); + let icon_with_format = match explicit_format { + Some(format) => { + icon.set_format(format); + icon + } + None => icon.with_guessed_format()?, + }; - let pixels = icon_with_format.decode()?.to_rgba8(); + let pixels = icon_with_format.decode()?.to_rgba8(); - Self::from_rgba(pixels.to_vec(), pixels.width(), pixels.height()) - } + Ok(icon::from_rgba( + pixels.to_vec(), + pixels.width(), + pixels.height(), + )?) } -/// An error produced when using `Icon::from_rgba` with invalid arguments. -#[derive(Debug)] +/// An error produced when creating an [`Icon`]. +#[derive(Debug, thiserror::Error)] pub enum Error { - /// The provided RGBA data isn't divisble by 4. - /// - /// Therefore, it cannot be safely interpreted as 32bpp RGBA pixels. - InvalidData { - /// The length of the provided RGBA data. - byte_count: usize, - }, - - /// The number of RGBA pixels does not match the provided dimensions. - DimensionsMismatch { - /// The provided width. - width: u32, - /// The provided height. - height: u32, - /// The amount of pixels of the provided RGBA data. - pixel_count: usize, - }, + /// The [`Icon`] is not valid. + #[error("The icon is invalid: {0}")] + InvalidError(#[from] icon::Error), /// The underlying OS failed to create the icon. - OsError(io::Error), - - /// The `image` crate reported an error - #[cfg(feature = "image_rs")] - ImageError(image_rs::error::ImageError), -} - -impl From<std::io::Error> for Error { - fn from(os_error: std::io::Error) -> Self { - Error::OsError(os_error) - } -} - -impl From<iced_winit::winit::window::BadIcon> for Error { - fn from(error: iced_winit::winit::window::BadIcon) -> Self { - use iced_winit::winit::window::BadIcon; - - match error { - BadIcon::ByteCountNotDivisibleBy4 { byte_count } => { - Error::InvalidData { byte_count } - } - BadIcon::DimensionsVsPixelCount { - width, - height, - pixel_count, - .. - } => Error::DimensionsMismatch { - width, - height, - pixel_count, - }, - BadIcon::OsError(os_error) => Error::OsError(os_error), - } - } -} - -impl From<Icon> for iced_winit::winit::window::Icon { - fn from(icon: Icon) -> Self { - icon.0 - } -} - -#[cfg(feature = "image_rs")] -impl From<image_rs::error::ImageError> for Error { - fn from(image_error: image_rs::error::ImageError) -> Self { - Self::ImageError(image_error) - } -} - -impl fmt::Display for Error { - fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result { - match self { - Error::InvalidData { byte_count } => { - write!( - f, - "The provided RGBA data (with length {byte_count:?}) isn't divisble by \ - 4. Therefore, it cannot be safely interpreted as 32bpp RGBA \ - pixels." - ) - } - Error::DimensionsMismatch { - width, - height, - pixel_count, - } => { - write!( - f, - "The number of RGBA pixels ({pixel_count:?}) does not match the provided \ - dimensions ({width:?}x{height:?})." - ) - } - Error::OsError(e) => write!( - f, - "The underlying OS failed to create the window \ - icon: {e:?}" - ), - #[cfg(feature = "image_rs")] - Error::ImageError(e) => { - write!(f, "Unable to create icon from a file: {e:?}") - } - } - } -} - -impl std::error::Error for Error { - fn source(&self) -> Option<&(dyn std::error::Error + 'static)> { - Some(self) - } + #[error("The underlying OS failted to create the window icon: {0}")] + OsError(#[from] io::Error), + + /// The `image` crate reported an error. + #[cfg(feature = "image")] + #[cfg_attr(docsrs, doc(cfg(feature = "image")))] + #[error("Unable to create icon from a file: {0}")] + ImageError(#[from] image_rs::error::ImageError), } diff --git a/style/src/slider.rs b/style/src/slider.rs index 4b52fad3..d5db1853 100644 --- a/style/src/slider.rs +++ b/style/src/slider.rs @@ -5,11 +5,22 @@ use iced_core::Color; #[derive(Debug, Clone, Copy)] pub struct Appearance { /// The colors of the rail of the slider. - pub rail_colors: (Color, Color), + pub rail: Rail, /// The appearance of the [`Handle`] of the slider. pub handle: Handle, } +/// The appearance of a slider rail +#[derive(Debug, Clone, Copy)] +pub struct Rail { + /// The colors of the rail of the slider. + pub colors: (Color, Color), + /// The width of the stroke of a slider rail. + pub width: f32, + /// The border radius of the slider. + pub border_radius: f32, +} + /// The appearance of the handle of a slider. #[derive(Debug, Clone, Copy)] pub struct Handle { diff --git a/style/src/text_input.rs b/style/src/text_input.rs index d97016dc..2616ad5a 100644 --- a/style/src/text_input.rs +++ b/style/src/text_input.rs @@ -12,6 +12,8 @@ pub struct Appearance { pub border_width: f32, /// The border [`Color`] of the text input. pub border_color: Color, + /// The icon [`Color`] of the text input. + pub icon_color: Color, } /// A set of rules that dictate the style of a text input. @@ -31,6 +33,9 @@ pub trait StyleSheet { /// Produces the [`Color`] of the value of a text input. fn value_color(&self, style: &Self::Style) -> Color; + /// Produces the [`Color`] of the value of a disabled text input. + fn disabled_color(&self, style: &Self::Style) -> Color; + /// Produces the [`Color`] of the selection of a text input. fn selection_color(&self, style: &Self::Style) -> Color; @@ -38,4 +43,7 @@ pub trait StyleSheet { fn hovered(&self, style: &Self::Style) -> Appearance { self.focused(style) } + + /// Produces the style of a disabled text input. + fn disabled(&self, style: &Self::Style) -> Appearance; } diff --git a/style/src/theme.rs b/style/src/theme.rs index 0ebd82a4..b195940e 100644 --- a/style/src/theme.rs +++ b/style/src/theme.rs @@ -416,10 +416,14 @@ impl slider::StyleSheet for Theme { }; slider::Appearance { - rail_colors: ( - palette.primary.base.color, - Color::TRANSPARENT, - ), + rail: slider::Rail { + colors: ( + palette.primary.base.color, + palette.primary.base.color, + ), + width: 2.0, + border_radius: 2.0, + }, handle: slider::Handle { color: palette.background.base.color, border_color: palette.primary.base.color, @@ -1028,6 +1032,7 @@ impl text_input::StyleSheet for Theme { border_radius: 2.0, border_width: 1.0, border_color: palette.background.strong.color, + icon_color: palette.background.weak.text, } } @@ -1043,6 +1048,7 @@ impl text_input::StyleSheet for Theme { border_radius: 2.0, border_width: 1.0, border_color: palette.background.base.text, + icon_color: palette.background.weak.text, } } @@ -1058,6 +1064,7 @@ impl text_input::StyleSheet for Theme { border_radius: 2.0, border_width: 1.0, border_color: palette.primary.strong.color, + icon_color: palette.background.weak.text, } } @@ -1090,4 +1097,28 @@ impl text_input::StyleSheet for Theme { palette.primary.weak.color } + + fn disabled(&self, style: &Self::Style) -> text_input::Appearance { + if let TextInput::Custom(custom) = style { + return custom.disabled(self); + } + + let palette = self.extended_palette(); + + text_input::Appearance { + background: palette.background.weak.color.into(), + border_radius: 2.0, + border_width: 1.0, + border_color: palette.background.strong.color, + icon_color: palette.background.strong.color, + } + } + + fn disabled_color(&self, style: &Self::Style) -> Color { + if let TextInput::Custom(custom) = style { + return custom.disabled_color(self); + } + + self.placeholder_color(style) + } } diff --git a/wgpu/src/image.rs b/wgpu/src/image.rs index a5e63b17..9f56c188 100644 --- a/wgpu/src/image.rs +++ b/wgpu/src/image.rs @@ -141,7 +141,7 @@ impl Pipeline { let shader = device.create_shader_module(wgpu::ShaderModuleDescriptor { - label: Some("iced_wgpu::image::shader"), + label: Some("iced_wgpu image shader"), source: wgpu::ShaderSource::Wgsl(std::borrow::Cow::Borrowed( include_str!("shader/image.wgsl"), )), diff --git a/wgpu/src/quad.rs b/wgpu/src/quad.rs index 2f5fcc6b..1343181e 100644 --- a/wgpu/src/quad.rs +++ b/wgpu/src/quad.rs @@ -63,7 +63,7 @@ impl Pipeline { let shader = device.create_shader_module(wgpu::ShaderModuleDescriptor { - label: Some("iced_wgpu::quad::shader"), + label: Some("iced_wgpu quad shader"), source: wgpu::ShaderSource::Wgsl(std::borrow::Cow::Borrowed( include_str!("shader/quad.wgsl"), )), diff --git a/wgpu/src/triangle.rs b/wgpu/src/triangle.rs index efdd214b..162428f0 100644 --- a/wgpu/src/triangle.rs +++ b/wgpu/src/triangle.rs @@ -457,7 +457,7 @@ mod solid { let shader = device.create_shader_module(wgpu::ShaderModuleDescriptor { label: Some( - "iced_wgpu::triangle::solid create shader module", + "iced_wgpu triangle solid create shader module", ), source: wgpu::ShaderSource::Wgsl( std::borrow::Cow::Borrowed(include_str!( @@ -654,7 +654,7 @@ mod gradient { let shader = device.create_shader_module(wgpu::ShaderModuleDescriptor { label: Some( - "iced_wgpu::triangle::gradient create shader module", + "iced_wgpu triangle gradient create shader module", ), source: wgpu::ShaderSource::Wgsl( std::borrow::Cow::Borrowed(include_str!( diff --git a/wgpu/src/triangle/msaa.rs b/wgpu/src/triangle/msaa.rs index a3016ff8..e76f7d5e 100644 --- a/wgpu/src/triangle/msaa.rs +++ b/wgpu/src/triangle/msaa.rs @@ -75,7 +75,7 @@ impl Blit { let shader = device.create_shader_module(wgpu::ShaderModuleDescriptor { - label: Some("iced_wgpu::triangle::blit_shader"), + label: Some("iced_wgpu triangle blit_shader"), source: wgpu::ShaderSource::Wgsl(std::borrow::Cow::Borrowed( include_str!("../shader/blit.wgsl"), )), diff --git a/winit/src/application.rs b/winit/src/application.rs index 31654f26..dd345785 100644 --- a/winit/src/application.rs +++ b/winit/src/application.rs @@ -770,6 +770,9 @@ pub fn run_command<A, E>( mode, )); } + window::Action::ChangeIcon(icon) => { + window.set_window_icon(conversion::icon(icon)) + } window::Action::FetchMode(tag) => { let mode = if window.is_visible().unwrap_or(true) { conversion::mode(window.fullscreen()) diff --git a/winit/src/conversion.rs b/winit/src/conversion.rs index 1b2ead36..cf066ef6 100644 --- a/winit/src/conversion.rs +++ b/winit/src/conversion.rs @@ -236,6 +236,7 @@ pub fn mouse_interaction( winit::window::CursorIcon::EwResize } Interaction::ResizingVertically => winit::window::CursorIcon::NsResize, + Interaction::NotAllowed => winit::window::CursorIcon::NotAllowed, } } @@ -509,6 +510,15 @@ pub fn user_attention( } } +/// Converts some [`Icon`] into it's `winit` counterpart. +/// +/// Returns `None` if there is an error during the conversion. +pub fn icon(icon: window::Icon) -> Option<winit::window::Icon> { + let (pixels, size) = icon.into_raw(); + + winit::window::Icon::from_rgba(pixels, size.width, size.height).ok() +} + // As defined in: http://www.unicode.org/faq/private_use.html pub(crate) fn is_private_use_character(c: char) -> bool { matches!( diff --git a/winit/src/settings.rs b/winit/src/settings.rs index 78d58000..6658773d 100644 --- a/winit/src/settings.rs +++ b/winit/src/settings.rs @@ -92,7 +92,7 @@ pub struct Window { pub always_on_top: bool, /// The window icon, which is also usually used in the taskbar - pub icon: Option<winit::window::Icon>, + pub icon: Option<crate::window::Icon>, /// Platform specific settings. pub platform_specific: platform::PlatformSpecific, @@ -134,8 +134,9 @@ impl Window { .with_resizable(self.resizable) .with_decorations(self.decorations) .with_transparent(self.transparent) - .with_window_icon(self.icon) - .with_always_on_top(self.always_on_top); + .with_window_icon(self.icon.and_then(conversion::icon)) + .with_always_on_top(self.always_on_top) + .with_visible(self.visible); if let Some(position) = conversion::position( primary_monitor.as_ref(), diff --git a/winit/src/window.rs b/winit/src/window.rs index 961562bd..ba0180c8 100644 --- a/winit/src/window.rs +++ b/winit/src/window.rs @@ -2,7 +2,9 @@ use crate::command::{self, Command}; use iced_native::window; -pub use window::{frames, Event, Mode, RedrawRequest, UserAttention}; +pub use window::{ + frames, icon, Event, Icon, Mode, RedrawRequest, UserAttention, +}; /// Closes the current window and exits the application. pub fn close<Message>() -> Command<Message> { @@ -104,3 +106,8 @@ pub fn fetch_id<Message>( f, )))) } + +/// Changes the [`Icon`] of the window. +pub fn change_icon<Message>(icon: Icon) -> Command<Message> { + Command::single(command::Action::Window(window::Action::ChangeIcon(icon))) +} |