diff options
author | 2023-03-04 05:37:11 +0100 | |
---|---|---|
committer | 2023-03-04 05:37:11 +0100 | |
commit | 3a0d34c0240f4421737a6a08761f99d6f8140d02 (patch) | |
tree | c9a4a6b8e9c1db1b8fcd05bc98e3f131d5ef4bd5 /native/src/widget/radio.rs | |
parent | c54409d1711e1f615c7ea4b02c082954e340632a (diff) | |
download | iced-3a0d34c0240f4421737a6a08761f99d6f8140d02.tar.gz iced-3a0d34c0240f4421737a6a08761f99d6f8140d02.tar.bz2 iced-3a0d34c0240f4421737a6a08761f99d6f8140d02.zip |
Create `iced_widget` subcrate and re-organize the whole codebase
Diffstat (limited to 'native/src/widget/radio.rs')
-rw-r--r-- | native/src/widget/radio.rs | 299 |
1 files changed, 0 insertions, 299 deletions
diff --git a/native/src/widget/radio.rs b/native/src/widget/radio.rs deleted file mode 100644 index 5f60eaef..00000000 --- a/native/src/widget/radio.rs +++ /dev/null @@ -1,299 +0,0 @@ -//! Create choices using radio buttons. -use crate::alignment; -use crate::event::{self, Event}; -use crate::layout; -use crate::mouse; -use crate::renderer; -use crate::text; -use crate::touch; -use crate::widget::{self, Row, Text, Tree}; -use crate::{ - Alignment, Clipboard, Color, Element, Layout, Length, Pixels, Point, - Rectangle, Shell, Widget, -}; - -pub use iced_style::radio::{Appearance, StyleSheet}; - -/// A circular button representing a choice. -/// -/// # Example -/// ``` -/// # type Radio<Message> = -/// # iced_native::widget::Radio<Message, iced_native::renderer::Null>; -/// # -/// #[derive(Debug, Clone, Copy, PartialEq, Eq)] -/// pub enum Choice { -/// A, -/// B, -/// } -/// -/// #[derive(Debug, Clone, Copy)] -/// pub enum Message { -/// RadioSelected(Choice), -/// } -/// -/// let selected_choice = Some(Choice::A); -/// -/// Radio::new(Choice::A, "This is A", selected_choice, Message::RadioSelected); -/// -/// Radio::new(Choice::B, "This is B", selected_choice, Message::RadioSelected); -/// ``` -/// -/// 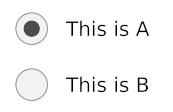 -#[allow(missing_debug_implementations)] -pub struct Radio<Message, Renderer> -where - Renderer: text::Renderer, - Renderer::Theme: StyleSheet, -{ - is_selected: bool, - on_click: Message, - label: String, - width: Length, - size: f32, - spacing: f32, - text_size: Option<f32>, - font: Option<Renderer::Font>, - style: <Renderer::Theme as StyleSheet>::Style, -} - -impl<Message, Renderer> Radio<Message, Renderer> -where - Message: Clone, - Renderer: text::Renderer, - Renderer::Theme: StyleSheet, -{ - /// The default size of a [`Radio`] button. - pub const DEFAULT_SIZE: f32 = 28.0; - - /// The default spacing of a [`Radio`] button. - pub const DEFAULT_SPACING: f32 = 15.0; - - /// Creates a new [`Radio`] button. - /// - /// It expects: - /// * the value related to the [`Radio`] button - /// * the label of the [`Radio`] button - /// * the current selected value - /// * a function that will be called when the [`Radio`] is selected. It - /// receives the value of the radio and must produce a `Message`. - pub fn new<F, V>( - value: V, - label: impl Into<String>, - selected: Option<V>, - f: F, - ) -> Self - where - V: Eq + Copy, - F: FnOnce(V) -> Message, - { - Radio { - is_selected: Some(value) == selected, - on_click: f(value), - label: label.into(), - width: Length::Shrink, - size: Self::DEFAULT_SIZE, - spacing: Self::DEFAULT_SPACING, //15 - text_size: None, - font: None, - style: Default::default(), - } - } - - /// Sets the size of the [`Radio`] button. - pub fn size(mut self, size: impl Into<Pixels>) -> Self { - self.size = size.into().0; - self - } - - /// Sets the width of the [`Radio`] button. - pub fn width(mut self, width: impl Into<Length>) -> Self { - self.width = width.into(); - self - } - - /// Sets the spacing between the [`Radio`] button and the text. - pub fn spacing(mut self, spacing: impl Into<Pixels>) -> Self { - self.spacing = spacing.into().0; - self - } - - /// Sets the text size of the [`Radio`] button. - pub fn text_size(mut self, text_size: impl Into<Pixels>) -> Self { - self.text_size = Some(text_size.into().0); - self - } - - /// Sets the text font of the [`Radio`] button. - pub fn font(mut self, font: impl Into<Renderer::Font>) -> Self { - self.font = Some(font.into()); - self - } - - /// Sets the style of the [`Radio`] button. - pub fn style( - mut self, - style: impl Into<<Renderer::Theme as StyleSheet>::Style>, - ) -> Self { - self.style = style.into(); - self - } -} - -impl<Message, Renderer> Widget<Message, Renderer> for Radio<Message, Renderer> -where - Message: Clone, - Renderer: text::Renderer, - Renderer::Theme: StyleSheet + widget::text::StyleSheet, -{ - fn width(&self) -> Length { - self.width - } - - fn height(&self) -> Length { - Length::Shrink - } - - fn layout( - &self, - renderer: &Renderer, - limits: &layout::Limits, - ) -> layout::Node { - Row::<(), Renderer>::new() - .width(self.width) - .spacing(self.spacing) - .align_items(Alignment::Center) - .push(Row::new().width(self.size).height(self.size)) - .push(Text::new(&self.label).width(self.width).size( - self.text_size.unwrap_or_else(|| renderer.default_size()), - )) - .layout(renderer, limits) - } - - fn on_event( - &mut self, - _state: &mut Tree, - event: Event, - layout: Layout<'_>, - cursor_position: Point, - _renderer: &Renderer, - _clipboard: &mut dyn Clipboard, - shell: &mut Shell<'_, Message>, - ) -> event::Status { - match event { - Event::Mouse(mouse::Event::ButtonPressed(mouse::Button::Left)) - | Event::Touch(touch::Event::FingerPressed { .. }) => { - if layout.bounds().contains(cursor_position) { - shell.publish(self.on_click.clone()); - - return event::Status::Captured; - } - } - _ => {} - } - - event::Status::Ignored - } - - fn mouse_interaction( - &self, - _state: &Tree, - layout: Layout<'_>, - cursor_position: Point, - _viewport: &Rectangle, - _renderer: &Renderer, - ) -> mouse::Interaction { - if layout.bounds().contains(cursor_position) { - mouse::Interaction::Pointer - } else { - mouse::Interaction::default() - } - } - - fn draw( - &self, - _state: &Tree, - renderer: &mut Renderer, - theme: &Renderer::Theme, - style: &renderer::Style, - layout: Layout<'_>, - cursor_position: Point, - _viewport: &Rectangle, - ) { - let bounds = layout.bounds(); - let is_mouse_over = bounds.contains(cursor_position); - - let mut children = layout.children(); - - let custom_style = if is_mouse_over { - theme.hovered(&self.style, self.is_selected) - } else { - theme.active(&self.style, self.is_selected) - }; - - { - let layout = children.next().unwrap(); - let bounds = layout.bounds(); - - let size = bounds.width; - let dot_size = size / 2.0; - - renderer.fill_quad( - renderer::Quad { - bounds, - border_radius: (size / 2.0).into(), - border_width: custom_style.border_width, - border_color: custom_style.border_color, - }, - custom_style.background, - ); - - if self.is_selected { - renderer.fill_quad( - renderer::Quad { - bounds: Rectangle { - x: bounds.x + dot_size / 2.0, - y: bounds.y + dot_size / 2.0, - width: bounds.width - dot_size, - height: bounds.height - dot_size, - }, - border_radius: (dot_size / 2.0).into(), - border_width: 0.0, - border_color: Color::TRANSPARENT, - }, - custom_style.dot_color, - ); - } - } - - { - let label_layout = children.next().unwrap(); - - widget::text::draw( - renderer, - style, - label_layout, - &self.label, - self.text_size, - self.font, - widget::text::Appearance { - color: custom_style.text_color, - }, - alignment::Horizontal::Left, - alignment::Vertical::Center, - ); - } - } -} - -impl<'a, Message, Renderer> From<Radio<Message, Renderer>> - for Element<'a, Message, Renderer> -where - Message: 'a + Clone, - Renderer: 'a + text::Renderer, - Renderer::Theme: StyleSheet + widget::text::StyleSheet, -{ - fn from(radio: Radio<Message, Renderer>) -> Element<'a, Message, Renderer> { - Element::new(radio) - } -} |