diff options
author | 2019-10-08 03:13:41 +0200 | |
---|---|---|
committer | 2019-10-08 03:13:41 +0200 | |
commit | 10e10e5e06841574425d2633f1c2916733f7b4ff (patch) | |
tree | 0407a0136973a201fe44bfc1a94268544a2f8eb5 /core | |
parent | a0234d5bcea5b25f575af01d3a8e0296b2d0395c (diff) | |
download | iced-10e10e5e06841574425d2633f1c2916733f7b4ff.tar.gz iced-10e10e5e06841574425d2633f1c2916733f7b4ff.tar.bz2 iced-10e10e5e06841574425d2633f1c2916733f7b4ff.zip |
Make `iced_core::Button` customizable
Now it supports:
- Any kind of content
- Custom border radius
- Custom background
Diffstat (limited to 'core')
-rw-r--r-- | core/src/background.rs | 7 | ||||
-rw-r--r-- | core/src/color.rs | 8 | ||||
-rw-r--r-- | core/src/lib.rs | 2 | ||||
-rw-r--r-- | core/src/widget/button.rs | 96 | ||||
-rw-r--r-- | core/src/widget/text.rs | 2 |
5 files changed, 52 insertions, 63 deletions
diff --git a/core/src/background.rs b/core/src/background.rs new file mode 100644 index 00000000..59b67a2c --- /dev/null +++ b/core/src/background.rs @@ -0,0 +1,7 @@ +use crate::Color; + +#[derive(Debug, Clone, Copy, PartialEq)] +pub enum Background { + Color(Color), + // TODO: Add gradient and image variants +} diff --git a/core/src/color.rs b/core/src/color.rs index 2b64c78d..79910dd8 100644 --- a/core/src/color.rs +++ b/core/src/color.rs @@ -17,6 +17,14 @@ impl Color { a: 1.0, }; + /// The white color. + pub const WHITE: Color = Color { + r: 1.0, + g: 1.0, + b: 1.0, + a: 1.0, + }; + pub fn into_linear(self) -> [f32; 4] { // As described in: // https://en.wikipedia.org/wiki/SRGB#The_reverse_transformation diff --git a/core/src/lib.rs b/core/src/lib.rs index 1f43b2b7..877a8f85 100644 --- a/core/src/lib.rs +++ b/core/src/lib.rs @@ -1,6 +1,7 @@ pub mod widget; mod align; +mod background; mod color; mod justify; mod length; @@ -9,6 +10,7 @@ mod rectangle; mod vector; pub use align::Align; +pub use background::Background; pub use color::Color; pub use justify::Justify; pub use length::Length; diff --git a/core/src/widget/button.rs b/core/src/widget/button.rs index b98bb443..a57f2dd8 100644 --- a/core/src/widget/button.rs +++ b/core/src/widget/button.rs @@ -5,68 +5,58 @@ //! [`Button`]: struct.Button.html //! [`State`]: struct.State.html -use crate::{Align, Length}; +use crate::{Align, Background, Length}; /// A generic widget that produces a message when clicked. -/// -/// # Example -/// -/// ``` -/// use iced_core::{button, Button}; -/// -/// pub enum Message { -/// ButtonClicked, -/// } -/// -/// let state = &mut button::State::new(); -/// -/// Button::new(state, "Click me!") -/// .on_press(Message::ButtonClicked); -/// ``` -/// -///  -pub struct Button<'a, Message> { +pub struct Button<'a, Message, Element> { /// The current state of the button pub state: &'a mut State, - /// The label of the button - pub label: String, + pub content: Element, /// The message to produce when the button is pressed pub on_press: Option<Message>, - pub class: Class, - pub width: Length, + pub padding: u16, + + pub background: Option<Background>, + + pub border_radius: u16, + pub align_self: Option<Align>, } -impl<'a, Message> std::fmt::Debug for Button<'a, Message> +impl<'a, Message, Element> std::fmt::Debug for Button<'a, Message, Element> where Message: std::fmt::Debug, { fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { f.debug_struct("Button") .field("state", &self.state) - .field("label", &self.label) .field("on_press", &self.on_press) .finish() } } -impl<'a, Message> Button<'a, Message> { +impl<'a, Message, Element> Button<'a, Message, Element> { /// Creates a new [`Button`] with some local [`State`] and the given label. /// /// [`Button`]: struct.Button.html /// [`State`]: struct.State.html - pub fn new(state: &'a mut State, label: &str) -> Self { + pub fn new<E>(state: &'a mut State, content: E) -> Self + where + E: Into<Element>, + { Button { state, - label: String::from(label), + content: content.into(), on_press: None, - class: Class::Primary, width: Length::Shrink, + padding: 0, + background: None, + border_radius: 0, align_self: None, } } @@ -79,6 +69,21 @@ impl<'a, Message> Button<'a, Message> { self } + pub fn padding(mut self, padding: u16) -> Self { + self.padding = padding; + self + } + + pub fn background(mut self, background: Background) -> Self { + self.background = Some(background); + self + } + + pub fn border_radius(mut self, border_radius: u16) -> Self { + self.border_radius = border_radius; + self + } + /// Sets the alignment of the [`Button`] itself. /// /// This is useful if you want to override the default alignment given by @@ -90,16 +95,6 @@ impl<'a, Message> Button<'a, Message> { self } - /// Sets the [`Class`] of the [`Button`]. - /// - /// - /// [`Button`]: struct.Button.html - /// [`Class`]: enum.Class.html - pub fn class(mut self, class: Class) -> Self { - self.class = class; - self - } - /// Sets the message that will be produced when the [`Button`] is pressed. /// /// [`Button`]: struct.Button.html @@ -133,26 +128,3 @@ impl State { self.is_pressed } } - -/// The type of a [`Button`]. -/// -/// 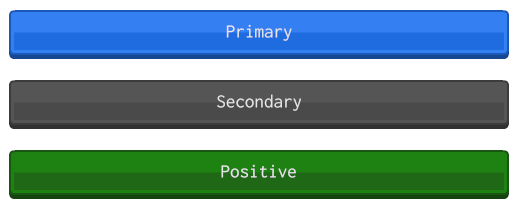 -/// -/// [`Button`]: struct.Button.html -#[derive(Debug, Clone, Copy, PartialEq, Eq)] -pub enum Class { - /// The [`Button`] performs the main action. - /// - /// [`Button`]: struct.Button.html - Primary, - - /// The [`Button`] performs an alternative action. - /// - /// [`Button`]: struct.Button.html - Secondary, - - /// The [`Button`] performs a productive action. - /// - /// [`Button`]: struct.Button.html - Positive, -} diff --git a/core/src/widget/text.rs b/core/src/widget/text.rs index 427d9471..cd94dbb2 100644 --- a/core/src/widget/text.rs +++ b/core/src/widget/text.rs @@ -1,5 +1,5 @@ //! Write some text for your users to read. -use crate::{Color, Length}; +use crate::{Align, Color, Length}; /// A paragraph of text. /// |