diff options
author | 2019-11-24 11:34:30 +0100 | |
---|---|---|
committer | 2019-11-24 11:34:30 +0100 | |
commit | 149fd2aa1fa86858c7c1dcec8fd844caa78cec94 (patch) | |
tree | a199cf8d2caaf6aa60e48e93d6dd0688969d43b0 /core | |
parent | 9712b319bb7a32848001b96bd84977430f14b623 (diff) | |
parent | 47196c9007d12d3b3e0036ffabe3bf6d14ff4523 (diff) | |
download | iced-149fd2aa1fa86858c7c1dcec8fd844caa78cec94.tar.gz iced-149fd2aa1fa86858c7c1dcec8fd844caa78cec94.tar.bz2 iced-149fd2aa1fa86858c7c1dcec8fd844caa78cec94.zip |
Merge pull request #65 from hecrj/improvement/docs
Documentation
Diffstat (limited to '')
-rw-r--r-- | core/src/align.rs | 40 | ||||
-rw-r--r-- | core/src/background.rs | 2 | ||||
-rw-r--r-- | core/src/color.rs | 3 | ||||
-rw-r--r-- | core/src/command.rs | 23 | ||||
-rw-r--r-- | core/src/font.rs | 10 | ||||
-rw-r--r-- | core/src/length.rs | 12 | ||||
-rw-r--r-- | core/src/lib.rs | 30 | ||||
-rw-r--r-- | core/src/vector.rs | 7 | ||||
-rw-r--r-- | core/src/widget.rs | 39 | ||||
-rw-r--r-- | core/src/widget/button.rs | 124 | ||||
-rw-r--r-- | core/src/widget/checkbox.rs | 78 | ||||
-rw-r--r-- | core/src/widget/column.rs | 125 | ||||
-rw-r--r-- | core/src/widget/container.rs | 84 | ||||
-rw-r--r-- | core/src/widget/image.rs | 65 | ||||
-rw-r--r-- | core/src/widget/radio.rs | 88 | ||||
-rw-r--r-- | core/src/widget/row.rs | 120 | ||||
-rw-r--r-- | core/src/widget/scrollable.rs | 140 | ||||
-rw-r--r-- | core/src/widget/slider.rs | 123 | ||||
-rw-r--r-- | core/src/widget/text.rs | 126 | ||||
-rw-r--r-- | core/src/widget/text_input.rs | 156 |
20 files changed, 110 insertions, 1285 deletions
diff --git a/core/src/align.rs b/core/src/align.rs index d6915086..8a59afa1 100644 --- a/core/src/align.rs +++ b/core/src/align.rs @@ -1,18 +1,38 @@ -/// Alignment on the cross axis of a container. -/// -/// * On a [`Column`], it describes __horizontal__ alignment. -/// * On a [`Row`], it describes __vertical__ alignment. -/// -/// [`Column`]: widget/struct.Column.html -/// [`Row`]: widget/struct.Row.html +/// Alignment on an axis of a container. #[derive(Debug, Clone, Copy, PartialEq, Eq, Hash)] pub enum Align { - /// Align at the start of the cross axis. + /// Align at the start of the axis. Start, - /// Align at the center of the cross axis. + /// Align at the center of the axis. Center, - /// Align at the end of the cross axis. + /// Align at the end of the axis. End, } + +/// The horizontal alignment of some resource. +#[derive(Debug, Clone, Copy, PartialEq, Eq)] +pub enum HorizontalAlignment { + /// Align left + Left, + + /// Horizontally centered + Center, + + /// Align right + Right, +} + +/// The vertical alignment of some resource. +#[derive(Debug, Clone, Copy, PartialEq, Eq)] +pub enum VerticalAlignment { + /// Align top + Top, + + /// Vertically centered + Center, + + /// Align bottom + Bottom, +} diff --git a/core/src/background.rs b/core/src/background.rs index 59b67a2c..98047172 100644 --- a/core/src/background.rs +++ b/core/src/background.rs @@ -1,7 +1,9 @@ use crate::Color; +/// The background of some element. #[derive(Debug, Clone, Copy, PartialEq)] pub enum Background { + /// A solid color Color(Color), // TODO: Add gradient and image variants } diff --git a/core/src/color.rs b/core/src/color.rs index 150bd05b..ec48c185 100644 --- a/core/src/color.rs +++ b/core/src/color.rs @@ -25,6 +25,9 @@ impl Color { a: 1.0, }; + /// Converts the [`Color`] into its linear values. + /// + /// [`Color`]: struct.Color.html pub fn into_linear(self) -> [f32; 4] { // As described in: // https://en.wikipedia.org/wiki/SRGB#The_reverse_transformation diff --git a/core/src/command.rs b/core/src/command.rs index e1c865dd..14b48b5b 100644 --- a/core/src/command.rs +++ b/core/src/command.rs @@ -1,16 +1,30 @@ use futures::future::{BoxFuture, Future, FutureExt}; +/// A collection of async operations. +/// +/// You should be able to turn a future easily into a [`Command`], either by +/// using the `From` trait or [`Command::perform`]. +/// +/// [`Command`]: struct.Command.html pub struct Command<T> { futures: Vec<BoxFuture<'static, T>>, } impl<T> Command<T> { + /// Creates an empty [`Command`]. + /// + /// In other words, a [`Command`] that does nothing. + /// + /// [`Command`]: struct.Command.html pub fn none() -> Self { Self { futures: Vec::new(), } } + /// Creates a [`Command`] that performs the action of the given future. + /// + /// [`Command`]: struct.Command.html pub fn perform<A>( future: impl Future<Output = T> + 'static + Send, f: impl Fn(T) -> A + 'static + Send, @@ -20,12 +34,21 @@ impl<T> Command<T> { } } + /// Creates a [`Command`] that performs the actions of all the givens + /// futures. + /// + /// Once this command is run, all the futures will be exectued at once. + /// + /// [`Command`]: struct.Command.html pub fn batch(commands: impl Iterator<Item = Command<T>>) -> Self { Self { futures: commands.flat_map(|command| command.futures).collect(), } } + /// Converts a [`Command`] into its underlying list of futures. + /// + /// [`Command`]: struct.Command.html pub fn futures(self) -> Vec<BoxFuture<'static, T>> { self.futures } diff --git a/core/src/font.rs b/core/src/font.rs index 75ba6a72..be49c825 100644 --- a/core/src/font.rs +++ b/core/src/font.rs @@ -1,8 +1,18 @@ +/// A font. #[derive(Debug, Clone, Copy)] pub enum Font { + /// The default font. + /// + /// This is normally a font configured in a renderer or loaded from the + /// system. Default, + + /// An external font. External { + /// The name of the external font name: &'static str, + + /// The bytes of the external font bytes: &'static [u8], }, } diff --git a/core/src/length.rs b/core/src/length.rs index 73c227d8..63ba6207 100644 --- a/core/src/length.rs +++ b/core/src/length.rs @@ -1,12 +1,24 @@ /// The strategy used to fill space in a specific dimension. #[derive(Debug, Clone, Copy, PartialEq, Hash)] pub enum Length { + /// Fill all the remaining space Fill, + + /// Fill the least amount of space Shrink, + + /// Fill a fixed amount of space Units(u16), } impl Length { + /// Returns the _fill factor_ of the [`Length`]. + /// + /// The _fill factor_ is a relative unit describing how much of the + /// remaining space should be filled when compared to other elements. It + /// is only meant to be used by layout engines. + /// + /// [`Length`]: enum.Length.html pub fn fill_factor(&self) -> u16 { match self { Length::Fill => 1, diff --git a/core/src/lib.rs b/core/src/lib.rs index 3816f8a2..65304e8b 100644 --- a/core/src/lib.rs +++ b/core/src/lib.rs @@ -1,24 +1,40 @@ -pub mod widget; +//! The core library of [Iced]. +//! +//!  +//! +//! This library holds basic types that can be reused and re-exported in +//! different runtime implementations. For instance, both [`iced_native`] and +//! [`iced_web`] are built on top of `iced_core`. +//! +//! [Iced]: https://github.com/hecrj/iced +//! [`iced_native`]: https://github.com/hecrj/iced/tree/master/native +//! [`iced_web`]: https://github.com/hecrj/iced/tree/master/web +#![deny(missing_docs)] +#![deny(missing_debug_implementations)] +#![deny(unused_results)] +#![deny(unsafe_code)] +#![deny(rust_2018_idioms)] mod align; mod background; mod color; -#[cfg(feature = "command")] -mod command; mod font; mod length; mod point; mod rectangle; mod vector; -pub use align::Align; +pub use align::{Align, HorizontalAlignment, VerticalAlignment}; pub use background::Background; pub use color::Color; -#[cfg(feature = "command")] -pub use command::Command; pub use font::Font; pub use length::Length; pub use point::Point; pub use rectangle::Rectangle; pub use vector::Vector; -pub use widget::*; + +#[cfg(feature = "command")] +mod command; + +#[cfg(feature = "command")] +pub use command::Command; diff --git a/core/src/vector.rs b/core/src/vector.rs index 92bf64ff..7d87343a 100644 --- a/core/src/vector.rs +++ b/core/src/vector.rs @@ -1,7 +1,14 @@ /// A 2D vector. #[derive(Debug, Clone, Copy, PartialEq)] pub struct Vector<T = f32> { + /// The X component of the [`Vector`] + /// + /// [`Vector`]: struct.Vector.html pub x: T, + + /// The Y component of the [`Vector`] + /// + /// [`Vector`]: struct.Vector.html pub y: T, } diff --git a/core/src/widget.rs b/core/src/widget.rs deleted file mode 100644 index 9e629e4f..00000000 --- a/core/src/widget.rs +++ /dev/null @@ -1,39 +0,0 @@ -//! Use the essential widgets. -//! -//! # Re-exports -//! For convenience, the contents of this module are available at the root -//! module. Therefore, you can directly type: -//! -//! ``` -//! use iced_core::{button, Button}; -//! ``` -mod checkbox; -mod column; -mod container; -mod image; -mod radio; -mod row; - -pub mod button; -pub mod scrollable; -pub mod slider; -pub mod text; -pub mod text_input; - -#[doc(no_inline)] -pub use button::Button; -#[doc(no_inline)] -pub use scrollable::Scrollable; -#[doc(no_inline)] -pub use slider::Slider; -#[doc(no_inline)] -pub use text::Text; -#[doc(no_inline)] -pub use text_input::TextInput; - -pub use checkbox::Checkbox; -pub use column::Column; -pub use container::Container; -pub use image::Image; -pub use radio::Radio; -pub use row::Row; diff --git a/core/src/widget/button.rs b/core/src/widget/button.rs deleted file mode 100644 index e7961284..00000000 --- a/core/src/widget/button.rs +++ /dev/null @@ -1,124 +0,0 @@ -//! Allow your users to perform actions by pressing a button. -//! -//! A [`Button`] has some local [`State`]. -//! -//! [`Button`]: struct.Button.html -//! [`State`]: struct.State.html - -use crate::{Background, Length}; - -/// A generic widget that produces a message when clicked. -pub struct Button<'a, Message, Element> { - /// The current state of the button - pub state: &'a mut State, - - pub content: Element, - - /// The message to produce when the button is pressed - pub on_press: Option<Message>, - - pub width: Length, - - pub min_width: u32, - - pub padding: u16, - - pub background: Option<Background>, - - pub border_radius: u16, -} - -impl<'a, Message, Element> std::fmt::Debug for Button<'a, Message, Element> -where - Message: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Button") - .field("state", &self.state) - .field("on_press", &self.on_press) - .finish() - } -} - -impl<'a, Message, Element> Button<'a, Message, Element> { - /// Creates a new [`Button`] with some local [`State`] and the given label. - /// - /// [`Button`]: struct.Button.html - /// [`State`]: struct.State.html - pub fn new<E>(state: &'a mut State, content: E) -> Self - where - E: Into<Element>, - { - Button { - state, - content: content.into(), - on_press: None, - width: Length::Shrink, - min_width: 0, - padding: 0, - background: None, - border_radius: 0, - } - } - - /// Sets the width of the [`Button`]. - /// - /// [`Button`]: struct.Button.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - pub fn min_width(mut self, min_width: u32) -> Self { - self.min_width = min_width; - self - } - - pub fn padding(mut self, padding: u16) -> Self { - self.padding = padding; - self - } - - pub fn background(mut self, background: Background) -> Self { - self.background = Some(background); - self - } - - pub fn border_radius(mut self, border_radius: u16) -> Self { - self.border_radius = border_radius; - self - } - - /// Sets the message that will be produced when the [`Button`] is pressed. - /// - /// [`Button`]: struct.Button.html - pub fn on_press(mut self, msg: Message) -> Self { - self.on_press = Some(msg); - self - } -} - -/// The local state of a [`Button`]. -/// -/// [`Button`]: struct.Button.html -#[derive(Debug, Clone, Copy, PartialEq, Eq, Default)] -pub struct State { - pub is_pressed: bool, -} - -impl State { - /// Creates a new [`State`]. - /// - /// [`State`]: struct.State.html - pub fn new() -> State { - State::default() - } - - /// Returns whether the associated [`Button`] is currently being pressed or - /// not. - /// - /// [`Button`]: struct.Button.html - pub fn is_pressed(&self) -> bool { - self.is_pressed - } -} diff --git a/core/src/widget/checkbox.rs b/core/src/widget/checkbox.rs deleted file mode 100644 index 1f0a0c04..00000000 --- a/core/src/widget/checkbox.rs +++ /dev/null @@ -1,78 +0,0 @@ -//! Show toggle controls using checkboxes. -use crate::Color; - -/// A box that can be checked. -/// -/// # Example -/// -/// ``` -/// use iced_core::Checkbox; -/// -/// pub enum Message { -/// CheckboxToggled(bool), -/// } -/// -/// let is_checked = true; -/// -/// Checkbox::new(is_checked, "Toggle me!", Message::CheckboxToggled); -/// ``` -/// -/// 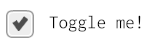 -pub struct Checkbox<Message> { - /// Whether the checkbox is checked or not - pub is_checked: bool, - - /// Function to call when checkbox is toggled to produce a __message__. - /// - /// The function should be provided `true` when the checkbox is checked - /// and `false` otherwise. - pub on_toggle: Box<dyn Fn(bool) -> Message>, - - /// The label of the checkbox - pub label: String, - - /// The color of the label - pub label_color: Option<Color>, -} - -impl<Message> std::fmt::Debug for Checkbox<Message> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Checkbox") - .field("is_checked", &self.is_checked) - .field("label", &self.label) - .field("label_color", &self.label_color) - .finish() - } -} - -impl<Message> Checkbox<Message> { - /// Creates a new [`Checkbox`]. - /// - /// It expects: - /// * a boolean describing whether the [`Checkbox`] is checked or not - /// * the label of the [`Checkbox`] - /// * a function that will be called when the [`Checkbox`] is toggled. - /// It will receive the new state of the [`Checkbox`] and must produce - /// a `Message`. - /// - /// [`Checkbox`]: struct.Checkbox.html - pub fn new<F>(is_checked: bool, label: &str, f: F) -> Self - where - F: 'static + Fn(bool) -> Message, - { - Checkbox { - is_checked, - on_toggle: Box::new(f), - label: String::from(label), - label_color: None, - } - } - - /// Sets the color of the label of the [`Checkbox`]. - /// - /// [`Checkbox`]: struct.Checkbox.html - pub fn label_color<C: Into<Color>>(mut self, color: C) -> Self { - self.label_color = Some(color.into()); - self - } -} diff --git a/core/src/widget/column.rs b/core/src/widget/column.rs deleted file mode 100644 index 4a97ad98..00000000 --- a/core/src/widget/column.rs +++ /dev/null @@ -1,125 +0,0 @@ -use crate::{Align, Length}; - -use std::u32; - -/// A container that distributes its contents vertically. -/// -/// A [`Column`] will try to fill the horizontal space of its container. -/// -/// [`Column`]: struct.Column.html -pub struct Column<Element> { - pub spacing: u16, - pub padding: u16, - pub width: Length, - pub height: Length, - pub max_width: u32, - pub max_height: u32, - pub align_items: Align, - pub children: Vec<Element>, -} - -impl<Element> Column<Element> { - /// Creates an empty [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn new() -> Self { - Column { - spacing: 0, - padding: 0, - width: Length::Fill, - height: Length::Shrink, - max_width: u32::MAX, - max_height: u32::MAX, - align_items: Align::Start, - children: Vec::new(), - } - } - - /// Sets the vertical spacing _between_ elements. - /// - /// Custom margins per element do not exist in Iced. You should use this - /// method instead! While less flexible, it helps you keep spacing between - /// elements consistent. - pub fn spacing(mut self, units: u16) -> Self { - self.spacing = units; - self - } - - /// Sets the padding of the [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn padding(mut self, units: u16) -> Self { - self.padding = units; - self - } - - /// Sets the width of the [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - /// Sets the height of the [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn height(mut self, height: Length) -> Self { - self.height = height; - self - } - - /// Sets the maximum width of the [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn max_width(mut self, max_width: u32) -> Self { - self.max_width = max_width; - self - } - - /// Sets the maximum height of the [`Column`] in pixels. - /// - /// [`Column`]: struct.Column.html - pub fn max_height(mut self, max_height: u32) -> Self { - self.max_height = max_height; - self - } - - /// Sets the horizontal alignment of the contents of the [`Column`] . - /// - /// [`Column`]: struct.Column.html - pub fn align_items(mut self, align: Align) -> Self { - self.align_items = align; - self - } - - /// Adds an element to the [`Column`]. - /// - /// [`Column`]: struct.Column.html - pub fn push<E>(mut self, child: E) -> Column<Element> - where - E: Into<Element>, - { - self.children.push(child.into()); - self - } -} - -impl<Element> Default for Column<Element> { - fn default() -> Self { - Self::new() - } -} - -impl<Element> std::fmt::Debug for Column<Element> -where - Element: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - // TODO: Complete once stabilized - f.debug_struct("Column") - .field("spacing", &self.spacing) - .field("children", &self.children) - .finish() - } -} diff --git a/core/src/widget/container.rs b/core/src/widget/container.rs deleted file mode 100644 index 9bc92fe0..00000000 --- a/core/src/widget/container.rs +++ /dev/null @@ -1,84 +0,0 @@ -use crate::{Align, Length}; - -use std::u32; - -#[derive(Debug)] -pub struct Container<Element> { - pub width: Length, - pub height: Length, - pub max_width: u32, - pub max_height: u32, - pub horizontal_alignment: Align, - pub vertical_alignment: Align, - pub content: Element, -} - -impl<Element> Container<Element> { - /// Creates an empty [`Container`]. - /// - /// [`Container`]: struct.Container.html - pub fn new<T>(content: T) -> Self - where - T: Into<Element>, - { - Container { - width: Length::Shrink, - height: Length::Shrink, - max_width: u32::MAX, - max_height: u32::MAX, - horizontal_alignment: Align::Start, - vertical_alignment: Align::Start, - content: content.into(), - } - } - - /// Sets the width of the [`Container`]. - /// - /// [`Container`]: struct.Container.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - /// Sets the height of the [`Container`]. - /// - /// [`Container`]: struct.Container.html - pub fn height(mut self, height: Length) -> Self { - self.height = height; - self - } - - /// Sets the maximum width of the [`Container`]. - /// - /// [`Container`]: struct.Container.html - pub fn max_width(mut self, max_width: u32) -> Self { - self.max_width = max_width; - self - } - - /// Sets the maximum height of the [`Container`] in pixels. - /// - /// [`Container`]: struct.Container.html - pub fn max_height(mut self, max_height: u32) -> Self { - self.max_height = max_height; - self - } - - /// Centers the contents in the horizontal axis of the [`Container`]. - /// - /// [`Container`]: struct.Container.html - pub fn center_x(mut self) -> Self { - self.horizontal_alignment = Align::Center; - - self - } - - /// Centers the contents in the vertical axis of the [`Container`]. - /// - /// [`Container`]: struct.Container.html - pub fn center_y(mut self) -> Self { - self.vertical_alignment = Align::Center; - - self - } -} diff --git a/core/src/widget/image.rs b/core/src/widget/image.rs deleted file mode 100644 index 996ab5e1..00000000 --- a/core/src/widget/image.rs +++ /dev/null @@ -1,65 +0,0 @@ -//! Display images in your user interface. - -use crate::{Length, Rectangle}; - -/// A frame that displays an image while keeping aspect ratio. -/// -/// # Example -/// -/// ``` -/// use iced_core::Image; -/// -/// let image = Image::new("resources/ferris.png"); -/// ``` -#[derive(Debug)] -pub struct Image { - /// The image path - pub path: String, - - /// The part of the image to show - pub clip: Option<Rectangle<u16>>, - - /// The width of the image - pub width: Length, - - /// The height of the image - pub height: Length, -} - -impl Image { - /// Creates a new [`Image`] with the given path. - /// - /// [`Image`]: struct.Image.html - pub fn new<T: Into<String>>(path: T) -> Self { - Image { - path: path.into(), - clip: None, - width: Length::Shrink, - height: Length::Shrink, - } - } - - /// Sets the portion of the [`Image`] to draw. - /// - /// [`Image`]: struct.Image.html - pub fn clip(mut self, clip: Rectangle<u16>) -> Self { - self.clip = Some(clip); - self - } - - /// Sets the width of the [`Image`] boundaries. - /// - /// [`Image`]: struct.Image.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - /// Sets the height of the [`Image`] boundaries. - /// - /// [`Image`]: struct.Image.html - pub fn height(mut self, height: Length) -> Self { - self.height = height; - self - } -} diff --git a/core/src/widget/radio.rs b/core/src/widget/radio.rs deleted file mode 100644 index 9765e928..00000000 --- a/core/src/widget/radio.rs +++ /dev/null @@ -1,88 +0,0 @@ -//! Create choices using radio buttons. -use crate::Color; - -/// A circular button representing a choice. -/// -/// # Example -/// ``` -/// use iced_core::Radio; -/// -/// #[derive(Debug, Clone, Copy, PartialEq, Eq)] -/// pub enum Choice { -/// A, -/// B, -/// } -/// -/// #[derive(Debug, Clone, Copy)] -/// pub enum Message { -/// RadioSelected(Choice), -/// } -/// -/// let selected_choice = Some(Choice::A); -/// -/// Radio::new(Choice::A, "This is A", selected_choice, Message::RadioSelected); -/// -/// Radio::new(Choice::B, "This is B", selected_choice, Message::RadioSelected); -/// ``` -/// -/// 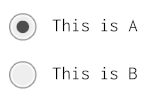 -pub struct Radio<Message> { - /// Whether the radio button is selected or not - pub is_selected: bool, - - /// The message to produce when the radio button is clicked - pub on_click: Message, - - /// The label of the radio button - pub label: String, - - /// The color of the label - pub label_color: Option<Color>, -} - -impl<Message> std::fmt::Debug for Radio<Message> -where - Message: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Radio") - .field("is_selected", &self.is_selected) - .field("on_click", &self.on_click) - .field("label", &self.label) - .field("label_color", &self.label_color) - .finish() - } -} - -impl<Message> Radio<Message> { - /// Creates a new [`Radio`] button. - /// - /// It expects: - /// * the value related to the [`Radio`] button - /// * the label of the [`Radio`] button - /// * the current selected value - /// * a function that will be called when the [`Radio`] is selected. It - /// receives the value of the radio and must produce a `Message`. - /// - /// [`Radio`]: struct.Radio.html - pub fn new<F, V>(value: V, label: &str, selected: Option<V>, f: F) -> Self - where - V: Eq + Copy, - F: 'static + Fn(V) -> Message, - { - Radio { - is_selected: Some(value) == selected, - on_click: f(value), - label: String::from(label), - label_color: None, - } - } - - /// Sets the `Color` of the label of the [`Radio`]. - /// - /// [`Radio`]: struct.Radio.html - pub fn label_color<C: Into<Color>>(mut self, color: C) -> Self { - self.label_color = Some(color.into()); - self - } -} diff --git a/core/src/widget/row.rs b/core/src/widget/row.rs deleted file mode 100644 index 3d882b47..00000000 --- a/core/src/widget/row.rs +++ /dev/null @@ -1,120 +0,0 @@ -use crate::{Align, Length}; - -use std::u32; - -/// A container that distributes its contents horizontally. -/// -/// A [`Row`] will try to fill the horizontal space of its container. -/// -/// [`Row`]: struct.Row.html -pub struct Row<Element> { - pub spacing: u16, - pub padding: u16, - pub width: Length, - pub height: Length, - pub max_width: u32, - pub max_height: u32, - pub align_items: Align, - pub children: Vec<Element>, -} - -impl<Element> Row<Element> { - /// Creates an empty [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn new() -> Self { - Row { - spacing: 0, - padding: 0, - width: Length::Fill, - height: Length::Shrink, - max_width: u32::MAX, - max_height: u32::MAX, - align_items: Align::Start, - children: Vec::new(), - } - } - - /// Sets the horizontal spacing _between_ elements. - /// - /// Custom margins per element do not exist in Iced. You should use this - /// method instead! While less flexible, it helps you keep spacing between - /// elements consistent. - pub fn spacing(mut self, units: u16) -> Self { - self.spacing = units; - self - } - - /// Sets the padding of the [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn padding(mut self, units: u16) -> Self { - self.padding = units; - self - } - - /// Sets the width of the [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - /// Sets the height of the [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn height(mut self, height: Length) -> Self { - self.height = height; - self - } - - /// Sets the maximum width of the [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn max_width(mut self, max_width: u32) -> Self { - self.max_width = max_width; - self - } - - /// Sets the maximum height of the [`Row`]. - /// - /// [`Row`]: struct.Row.html - pub fn max_height(mut self, max_height: u32) -> Self { - self.max_height = max_height; - self - } - - /// Sets the vertical alignment of the contents of the [`Row`] . - /// - /// [`Row`]: struct.Row.html - pub fn align_items(mut self, align: Align) -> Self { - self.align_items = align; - self - } - - /// Adds an [`Element`] to the [`Row`]. - /// - /// [`Element`]: ../struct.Element.html - /// [`Row`]: struct.Row.html - pub fn push<E>(mut self, child: E) -> Row<Element> - where - E: Into<Element>, - { - self.children.push(child.into()); - self - } -} - -impl<Element> std::fmt::Debug for Row<Element> -where - Element: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - // TODO: Complete once stabilized - f.debug_struct("Row") - .field("spacing", &self.spacing) - .field("children", &self.children) - .finish() - } -} diff --git a/core/src/widget/scrollable.rs b/core/src/widget/scrollable.rs deleted file mode 100644 index 7f2f0e99..00000000 --- a/core/src/widget/scrollable.rs +++ /dev/null @@ -1,140 +0,0 @@ -use crate::{Align, Column, Length, Point, Rectangle}; - -use std::u32; - -#[derive(Debug)] -pub struct Scrollable<'a, Element> { - pub state: &'a mut State, - pub height: Length, - pub max_height: u32, - pub content: Column<Element>, -} - -impl<'a, Element> Scrollable<'a, Element> { - pub fn new(state: &'a mut State) -> Self { - Scrollable { - state, - height: Length::Shrink, - max_height: u32::MAX, - content: Column::new(), - } - } - - /// Sets the vertical spacing _between_ elements. - /// - /// Custom margins per element do not exist in Iced. You should use this - /// method instead! While less flexible, it helps you keep spacing between - /// elements consistent. - pub fn spacing(mut self, units: u16) -> Self { - self.content = self.content.spacing(units); - self - } - - /// Sets the padding of the [`Scrollable`]. - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn padding(mut self, units: u16) -> Self { - self.content = self.content.padding(units); - self - } - - /// Sets the width of the [`Scrollable`]. - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn width(mut self, width: Length) -> Self { - self.content = self.content.width(width); - self - } - - /// Sets the height of the [`Scrollable`]. - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn height(mut self, height: Length) -> Self { - self.height = height; - self - } - - /// Sets the maximum width of the [`Scrollable`]. - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn max_width(mut self, max_width: u32) -> Self { - self.content = self.content.max_width(max_width); - self - } - - /// Sets the maximum height of the [`Scrollable`] in pixels. - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn max_height(mut self, max_height: u32) -> Self { - self.max_height = max_height; - self - } - - /// Sets the horizontal alignment of the contents of the [`Scrollable`] . - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn align_items(mut self, align_items: Align) -> Self { - self.content = self.content.align_items(align_items); - self - } - - /// Adds an element to the [`Scrollable`]. - /// - /// [`Scrollable`]: struct.Scrollable.html - pub fn push<E>(mut self, child: E) -> Scrollable<'a, Element> - where - E: Into<Element>, - { - self.content = self.content.push(child); - self - } -} - -#[derive(Debug, Clone, Copy, Default)] -pub struct State { - pub scrollbar_grabbed_at: Option<Point>, - offset: u32, -} - -impl State { - pub fn new() -> Self { - State::default() - } - - pub fn scroll( - &mut self, - delta_y: f32, - bounds: Rectangle, - content_bounds: Rectangle, - ) { - if bounds.height >= content_bounds.height { - return; - } - - self.offset = (self.offset as i32 - delta_y.round() as i32) - .max(0) - .min((content_bounds.height - bounds.height) as i32) - as u32; - } - - pub fn scroll_to( - &mut self, - percentage: f32, - bounds: Rectangle, - content_bounds: Rectangle, - ) { - self.offset = ((content_bounds.height - bounds.height) * percentage) - .max(0.0) as u32; - } - - pub fn offset(&self, bounds: Rectangle, content_bounds: Rectangle) -> u32 { - let hidden_content = - (content_bounds.height - bounds.height).max(0.0).round() as u32; - - self.offset.min(hidden_content) - } - - pub fn is_scrollbar_grabbed(&self) -> bool { - self.scrollbar_grabbed_at.is_some() - } -} diff --git a/core/src/widget/slider.rs b/core/src/widget/slider.rs deleted file mode 100644 index b65e3991..00000000 --- a/core/src/widget/slider.rs +++ /dev/null @@ -1,123 +0,0 @@ -//! Display an interactive selector of a single value from a range of values. -//! -//! A [`Slider`] has some local [`State`]. -//! -//! [`Slider`]: struct.Slider.html -//! [`State`]: struct.State.html -use crate::Length; - -use std::ops::RangeInclusive; -use std::rc::Rc; - -/// An horizontal bar and a handle that selects a single value from a range of -/// values. -/// -/// A [`Slider`] will try to fill the horizontal space of its container. -/// -/// [`Slider`]: struct.Slider.html -/// -/// # Example -/// ``` -/// use iced_core::{slider, Slider}; -/// -/// pub enum Message { -/// SliderChanged(f32), -/// } -/// -/// let state = &mut slider::State::new(); -/// let value = 50.0; -/// -/// Slider::new(state, 0.0..=100.0, value, Message::SliderChanged); -/// ``` -/// -///  -pub struct Slider<'a, Message> { - /// The state of the slider - pub state: &'a mut State, - - /// The range of the slider - pub range: RangeInclusive<f32>, - - /// The current value of the slider - pub value: f32, - - /// The function to produce messages on change - pub on_change: Rc<Box<dyn Fn(f32) -> Message>>, - - pub width: Length, -} - -impl<'a, Message> std::fmt::Debug for Slider<'a, Message> { - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - f.debug_struct("Slider") - .field("state", &self.state) - .field("range", &self.range) - .field("value", &self.value) - .finish() - } -} - -impl<'a, Message> Slider<'a, Message> { - /// Creates a new [`Slider`]. - /// - /// It expects: - /// * the local [`State`] of the [`Slider`] - /// * an inclusive range of possible values - /// * the current value of the [`Slider`] - /// * a function that will be called when the [`Slider`] is dragged. - /// It receives the new value of the [`Slider`] and must produce a - /// `Message`. - /// - /// [`Slider`]: struct.Slider.html - /// [`State`]: struct.State.html - pub fn new<F>( - state: &'a mut State, - range: RangeInclusive<f32>, - value: f32, - on_change: F, - ) -> Self - where - F: 'static + Fn(f32) -> Message, - { - Slider { - state, - value: value.max(*range.start()).min(*range.end()), - range, - on_change: Rc::new(Box::new(on_change)), - width: Length::Fill, - } - } - - /// Sets the width of the [`Slider`]. - /// - /// [`Slider`]: struct.Slider.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } -} - -/// The local state of a [`Slider`]. -/// -/// [`Slider`]: struct.Slider.html -#[derive(Debug, Clone, Copy, PartialEq, Eq, Default)] -pub struct State { - pub is_dragging: bool, -} - -impl State { - /// Creates a new [`State`]. - /// - /// [`State`]: struct.State.html - pub fn new() -> State { - State::default() - } - - /// Returns whether the associated [`Slider`] is currently being dragged or - /// not. - /// - /// [`Slider`]: struct.Slider.html - pub fn is_dragging(&self) -> bool { - self.is_dragging - } -} diff --git a/core/src/widget/text.rs b/core/src/widget/text.rs deleted file mode 100644 index 0996e7ff..00000000 --- a/core/src/widget/text.rs +++ /dev/null @@ -1,126 +0,0 @@ -//! Write some text for your users to read. -use crate::{Color, Font, Length}; - -/// A paragraph of text. -/// -/// # Example -/// -/// ``` -/// use iced_core::Text; -/// -/// Text::new("I <3 iced!") -/// .size(40); -/// ``` -#[derive(Debug, Clone)] -pub struct Text { - pub content: String, - pub size: Option<u16>, - pub color: Option<Color>, - pub font: Font, - pub width: Length, - pub height: Length, - pub horizontal_alignment: HorizontalAlignment, - pub vertical_alignment: VerticalAlignment, -} - -impl Text { - /// Create a new fragment of [`Text`] with the given contents. - /// - /// [`Text`]: struct.Text.html - pub fn new(label: &str) -> Self { - Text { - content: String::from(label), - size: None, - color: None, - font: Font::Default, - width: Length::Fill, - height: Length::Shrink, - horizontal_alignment: HorizontalAlignment::Left, - vertical_alignment: VerticalAlignment::Top, - } - } - - /// Sets the size of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - pub fn size(mut self, size: u16) -> Self { - self.size = Some(size); - self - } - - /// Sets the `Color` of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - pub fn color<C: Into<Color>>(mut self, color: C) -> Self { - self.color = Some(color.into()); - self - } - - pub fn font(mut self, font: Font) -> Self { - self.font = font; - self - } - - /// Sets the width of the [`Text`] boundaries. - /// - /// [`Text`]: struct.Text.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - /// Sets the height of the [`Text`] boundaries. - /// - /// [`Text`]: struct.Text.html - pub fn height(mut self, height: Length) -> Self { - self.height = height; - self - } - - /// Sets the [`HorizontalAlignment`] of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - /// [`HorizontalAlignment`]: enum.HorizontalAlignment.html - pub fn horizontal_alignment( - mut self, - alignment: HorizontalAlignment, - ) -> Self { - self.horizontal_alignment = alignment; - self - } - - /// Sets the [`VerticalAlignment`] of the [`Text`]. - /// - /// [`Text`]: struct.Text.html - /// [`VerticalAlignment`]: enum.VerticalAlignment.html - pub fn vertical_alignment(mut self, alignment: VerticalAlignment) -> Self { - self.vertical_alignment = alignment; - self - } -} - -/// The horizontal alignment of some resource. -#[derive(Debug, Clone, Copy, PartialEq, Eq)] -pub enum HorizontalAlignment { - /// Align left - Left, - - /// Horizontally centered - Center, - - /// Align right - Right, -} - -/// The vertical alignment of some resource. -#[derive(Debug, Clone, Copy, PartialEq, Eq)] -pub enum VerticalAlignment { - /// Align top - Top, - - /// Vertically centered - Center, - - /// Align bottom - Bottom, -} diff --git a/core/src/widget/text_input.rs b/core/src/widget/text_input.rs deleted file mode 100644 index 16c67954..00000000 --- a/core/src/widget/text_input.rs +++ /dev/null @@ -1,156 +0,0 @@ -use crate::Length; - -pub struct TextInput<'a, Message> { - pub state: &'a mut State, - pub placeholder: String, - pub value: Value, - pub width: Length, - pub max_width: Length, - pub padding: u16, - pub size: Option<u16>, - pub on_change: Box<dyn Fn(String) -> Message>, - pub on_submit: Option<Message>, -} - -impl<'a, Message> TextInput<'a, Message> { - pub fn new<F>( - state: &'a mut State, - placeholder: &str, - value: &str, - on_change: F, - ) -> Self - where - F: 'static + Fn(String) -> Message, - { - Self { - state, - placeholder: String::from(placeholder), - value: Value::new(value), - width: Length::Fill, - max_width: Length::Shrink, - padding: 0, - size: None, - on_change: Box::new(on_change), - on_submit: None, - } - } - - /// Sets the width of the [`TextInput`]. - /// - /// [`TextInput`]: struct.TextInput.html - pub fn width(mut self, width: Length) -> Self { - self.width = width; - self - } - - /// Sets the maximum width of the [`TextInput`]. - /// - /// [`TextInput`]: struct.TextInput.html - pub fn max_width(mut self, max_width: Length) -> Self { - self.max_width = max_width; - self - } - - /// Sets the padding of the [`TextInput`]. - /// - /// [`TextInput`]: struct.TextInput.html - pub fn padding(mut self, units: u16) -> Self { - self.padding = units; - self - } - - pub fn size(mut self, size: u16) -> Self { - self.size = Some(size); - self - } - - pub fn on_submit(mut self, message: Message) -> Self { - self.on_submit = Some(message); - self - } -} - -impl<'a, Message> std::fmt::Debug for TextInput<'a, Message> -where - Message: std::fmt::Debug, -{ - fn fmt(&self, f: &mut std::fmt::Formatter<'_>) -> std::fmt::Result { - // TODO: Complete once stabilized - f.debug_struct("TextInput").finish() - } -} - -#[derive(Debug, Default, Clone)] -pub struct State { - pub is_focused: bool, - cursor_position: usize, -} - -impl State { - pub fn new() -> Self { - Self::default() - } - - pub fn focused() -> Self { - use std::usize; - - Self { - is_focused: true, - cursor_position: usize::MAX, - } - } - - pub fn move_cursor_right(&mut self, value: &Value) { - let current = self.cursor_position(value); - - if current < value.len() { - self.cursor_position = current + 1; - } - } - - pub fn move_cursor_left(&mut self, value: &Value) { - let current = self.cursor_position(value); - - if current > 0 { - self.cursor_position = current - 1; - } - } - - pub fn move_cursor_to_end(&mut self, value: &Value) { - self.cursor_position = value.len(); - } - - pub fn cursor_position(&self, value: &Value) -> usize { - self.cursor_position.min(value.len()) - } -} - -// TODO: Use `unicode-segmentation` -pub struct Value(Vec<char>); - -impl Value { - pub fn new(string: &str) -> Self { - Self(string.chars().collect()) - } - - pub fn len(&self) -> usize { - self.0.len() - } - - pub fn until(&self, index: usize) -> Self { - Self(self.0[..index.min(self.len())].to_vec()) - } - - pub fn to_string(&self) -> String { - use std::iter::FromIterator; - String::from_iter(self.0.iter()) - } - - pub fn insert(&mut self, index: usize, c: char) { - self.0.insert(index, c); - } - - pub fn remove(&mut self, index: usize) { - self.0.remove(index); - } -} |